c++协程实现demo
时间: 2024-06-08 10:03:25 浏览: 11
C++协程是一种轻量级的线程,可以在单个线程中实现多个协程。C++20引入了协程库,其中包括协程原语和一些实用程序,使协程的使用变得更加容易。下面是一个简单的C++协程实现demo:
```c++
#include <iostream>
#include <coroutine>
struct Generator {
struct promise_type {
int current_value;
Generator get_return_object() {
return Generator(std::coroutine_handle<promise_type>::from_promise(*this));
}
auto initial_suspend() { return std::suspend_always{}; }
auto final_suspend() noexcept { return std::suspend_always{}; }
void unhandled_exception() {}
void return_void() {}
auto yield_value(int value) {
current_value = value;
return std::suspend_always{};
}
};
std::coroutine_handle<promise_type> coroutine;
Generator(std::coroutine_handle<promise_type> h) : coroutine(h) {}
~Generator() { if (coroutine) coroutine.destroy(); }
int get() {
coroutine.resume();
return coroutine.promise().current_value;
}
};
Generator counter(int begin, int end) {
for (int i = begin; i <= end; ++i)
co_yield i;
}
int main() {
auto gen = counter(1, 5);
while (auto value = gen.get())
std::cout << value << ' ';
}
```
这个demo中,我们定义了一个生成器(Generator)结构体和一个promise_type结构体。Generator结构体包含一个协程句柄,可以通过该句柄来操作协程。promise_type结构体定义了生成器的类型,并提供了返回对象,挂起,终止等方法。在counter函数中,我们使用co_yield关键字来挂起当前协程并返回值。在主函数中,我们使用while循环来不断调用协程的get方法来获取生成的值。
相关推荐
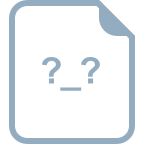
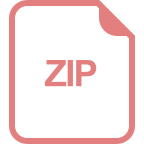
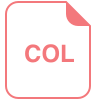
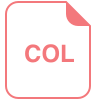
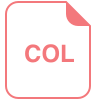
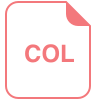
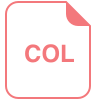









