用python写一个炸弹人小游戏的代码
时间: 2024-06-10 22:06:26 浏览: 5
好的,以下是炸弹人小游戏的Python代码:
```Python
import numpy as np
import os
import keyboard
# 游戏地图
map_array = np.array([
[1,1,1,1,1,1,1,1,1,1],
[1,0,0,0,1,0,0,0,0,1],
[1,0,1,0,1,0,1,1,0,1],
[1,0,1,0,0,0,0,0,0,1],
[1,0,1,1,1,0,1,1,0,1],
[1,0,0,0,0,0,0,0,0,1],
[1,0,1,1,1,0,1,1,0,1],
[1,0,0,0,1,0,0,0,0,1],
[1,0,1,0,1,0,1,0,0,1],
[1,1,1,1,1,1,1,1,1,1]
])
# 坐标类
class Coordinate(object):
def __init__(self, x, y):
self.x, self.y = x, y
# 角色类
class Character(object):
def __init__(self, x, y):
self.pos = Coordinate(x, y)
self.image = ""
self.speed = 0.5
self.direction = "right"
# 移动角色
def move(self, key):
if key == "up":
if map_array[self.pos.y-1, self.pos.x] == 0:
self.pos.y -= 1
elif key == "down":
if map_array[self.pos.y+1, self.pos.x] == 0:
self.pos.y += 1
elif key == "left":
if map_array[self.pos.y, self.pos.x-1] == 0:
self.pos.x -= 1
self.direction = "left"
elif key == "right":
if map_array[self.pos.y, self.pos.x+1] == 0:
self.pos.x += 1
self.direction = "right"
# 显示角色
def display(self):
os.system("cls")
for i in range(map_array.shape[0]):
for j in range(map_array.shape[1]):
if i == self.pos.y and j == self.pos.x:
print(self.image[self.direction], end=" ")
elif map_array[i, j] == 1:
print("X", end=" ")
else:
print(" ", end=" ")
print()
# 地图类
class Map(object):
# 显示地图
@staticmethod
def display():
for i in range(map_array.shape[0]):
for j in range(map_array.shape[1]):
if map_array[i, j] == 1:
print("X", end=" ")
else:
print(" ", end=" ")
print()
# 炸弹类
class Bomb(object):
def __init__(self, x, y):
self.pos = Coordinate(x, y)
self.timer = 3
# 显示炸弹
def display(self):
print("O", end=" ")
# 触发炸弹
def explode(self):
map_array[self.pos.y, self.pos.x] = 0
for i in range(1, 4):
if map_array[self.pos.y+i, self.pos.x] == 1:
map_array[self.pos.y+i, self.pos.x] = 0
else:
break
for i in range(1, 4):
if map_array[self.pos.y-i, self.pos.x] == 1:
map_array[self.pos.y-i, self.pos.x] = 0
else:
break
for i in range(1, 4):
if map_array[self.pos.y, self.pos.x+i] == 1:
map_array[self.pos.y, self.pos.x+i] = 0
else:
break
for i in range(1, 4):
if map_array[self.pos.y, self.pos.x-i] == 1:
map_array[self.pos.y, self.pos.x-i] = 0
else:
break
# 游戏主循环
def main():
player = Character(1, 1)
player.image = {"right":">", "left":"<"}
bomb_list = []
while True:
player.display()
key = ""
if keyboard.is_pressed("up"):
key = "up"
elif keyboard.is_pressed("down"):
key = "down"
elif keyboard.is_pressed("left"):
key = "left"
elif keyboard.is_pressed("right"):
key = "right"
elif keyboard.is_pressed("space"):
bomb_list.append(Bomb(player.pos.x, player.pos.y))
if len(bomb_list) > 0:
for bomb in bomb_list:
bomb.timer -= 1
if bomb.timer == 0:
bomb.explode()
bomb_list.remove(bomb)
if key != "":
player.move(key)
if __name__ == "__main__":
Map.display()
main()
```
希望对你有所帮助!
相关推荐
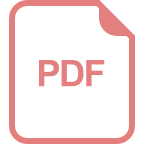















