qlineedit 点击后出现日历控件,日历控件点击日期会令qlineedit显示该日期,点击空白区域 日历控件隐藏,该qlineedit只能通过日历进行输入数据
时间: 2024-02-09 15:08:01 浏览: 158
您可以使用QCalendarWidget和QLineEdit来实现这个功能。您可以将QCalendarWidget放在一个QDialog中,并在QLineEdit中使用QLineEdit::setReadOnly(true)方法来防止用户直接在QLineEdit中输入数据。
当用户单击QLineEdit时,您可以显示这个QDialog,以便用户可以选择日期。当用户选择日期时,您可以使用QLineEdit::setText()方法来设置QLineEdit中的文本。另外,您可以使用QWidget::hide()方法来隐藏这个QDialog,当用户单击空白区域时。
以下是一个简单的代码示例,它实现了这个功能:
#include <QtWidgets>
class DateSelectionDialog : public QDialog
{
Q_OBJECT
public:
DateSelectionDialog(QWidget *parent = nullptr)
: QDialog(parent)
{
QVBoxLayout *layout = new QVBoxLayout(this);
m_calendar = new QCalendarWidget(this);
layout->addWidget(m_calendar);
connect(m_calendar, &QCalendarWidget::clicked, this, &DateSelectionDialog::onCalendarClicked);
}
QDate selectedDate() const
{
return m_selectedDate;
}
signals:
void dateSelected(const QDate &date);
private slots:
void onCalendarClicked(const QDate &date)
{
m_selectedDate = date;
emit dateSelected(date);
hide();
}
private:
QCalendarWidget *m_calendar;
QDate m_selectedDate;
};
class DateLineEdit : public QLineEdit
{
Q_OBJECT
public:
DateLineEdit(QWidget *parent = nullptr)
: QLineEdit(parent)
{
setReadOnly(true);
connect(this, &QLineEdit::clicked, this, &DateLineEdit::onClicked);
}
private slots:
void onClicked()
{
QPoint pos(0, height());
pos = mapToGlobal(pos);
m_dateSelectionDialog.move(pos);
m_dateSelectionDialog.show();
}
void onDateSelected(const QDate &date)
{
setText(date.toString(Qt::ISODate));
}
private:
DateSelectionDialog m_dateSelectionDialog;
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
DateLineEdit dateLineEdit;
dateLineEdit.show();
return app.exec();
}
#include "main.moc"
在这个代码示例中,我们创建了一个名为DateSelectionDialog的自定义对话框,它包含一个QCalendarWidget。当用户单击日期时,我们将所选日期存储在m_selectedDate成员变量中,然后使用dateSelected信号通知父窗口日期已经被选择。
我们还创建了一个名为DateLineEdit的自定义QLineEdit,它包含一个DateSelectionDialog对象。当用户单击QLineEdit时,我们将DateSelectionDialog移动到QLineEdit下方,并显示它。当用户选择日期时,我们使用setText()方法设置QLineEdit中的文本。另外,当用户单击空白区域时,DateSelectionDialog会自动隐藏。
相关推荐







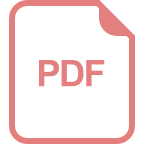










