c#如何在方法里比对泛型参数的类型
时间: 2024-12-11 09:40:17 浏览: 7
在 C# 中,如果你想在方法内部检查泛型参数的实际类型并进行相应的操作,可以使用 `as` 关键字或者类型断言 (`is`) 来尝试转换。以下是两种常见的做法:
1. **Using `as`关键字**:
```csharp
public void Process<T>(T value) where T : class
{
if (value is SomeSpecificType specificInstance)
{
// 这里你可以对specificInstance进行操作
specificInstance.Method();
}
else
{
// 如果不是预期类型,这里处理异常或默认情况
Console.WriteLine("Value is not of type SomeSpecificType.");
}
}
```
在这里,`SomeSpecificType`应该是 `T` 的一个子类型。
2. **使用类型断言 (`is` 和 `typeof`)**:
```csharp
public void Process<T>(T value) where T : class
{
var expectedType = typeof(SomeSpecificType);
if (value is SomeSpecificType specificInstance)
{
// 类似上面的操作
specificInstance.Method();
}
else if (expectedType.IsAssignableFrom(value.GetType()))
{
// 检查是否可以向下转换,而不是完全匹配
Console.WriteLine($"Value can be cast to {expectedType.Name}.");
}
else
{
Console.WriteLine("Value is not of type SomeSpecificType or its derived types.");
}
}
```
在这个例子中,`IsAssignableFrom()` 方法用于检查一个类型能否被赋值给另一个类型。
阅读全文
相关推荐
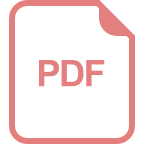
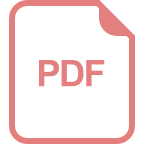
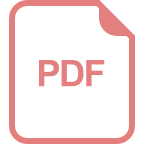















