假设输入一个正弦信号,如何利用c++通过编程生成李萨如图形,给出完整代码
时间: 2024-12-22 18:33:34 浏览: 3
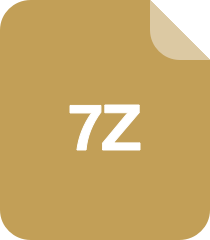
用VC绘制李萨如图形
要使用C++生成李萨如图形(Lissajous curve),你可以使用双线性插值法和基本图形绘制功能。这里提供一个简单的例子,假设你已经有一个函数`generate_sinusoidal_signal(double frequency, double amplitude)`用于生成给定频率和振幅的正弦波信号。
```cpp
#include <iostream>
#include <cmath>
#include <vector>
#include <SFML/Graphics.hpp> // 使用SFML库处理图形
// 生成正弦波信号
double generate_sinusoidal_signal(double t, double frequency, double amplitude) {
return amplitude * sin(2 * M_PI * frequency * t);
}
// 双线性插值函数
int interpolate(double x, double y0, double y1, double x0, double x1) {
return static_cast<int>(y0 + (y1 - y0) * (x - x0) / (x1 - x0));
}
void draw_lissajous_curve(int width, int height, double a1, double f1, double a2, double f2) {
sf::RenderWindow window(sf::VideoMode(width, height), "Lissajous Curve");
window.setFramerateLimit(60);
sf::Font font;
if (!font.loadFromFile("arial.ttf")) { // 加载字体文件
std::cout << "Failed to load font." << std::endl;
return;
}
for (int y = 0; y < height; ++y) {
for (int x = 0; x < width; ++x) {
double t1 = static_cast<double>(x) / window.getSize().x;
double t2 = static_cast<double>(y) / window.getSize().y;
double u1 = generate_sinusoidal_signal(t1, f1, a1);
double u2 = generate_sinusoidal_signal(t2, f2, a2);
sf::Vertex vertex(sf::Vector2f(x, y));
vertex.color = sf::Color(255, 255, 255, interpolate(u1, 0, 255, -1, 1) << 8 | interpolate(u2, 0, 255, -1, 1)); // 根据坐标转换颜色
window.draw(vertex);
}
}
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.display();
}
}
int main() {
const int screen_width = 800;
const int screen_height = 600;
double freq1 = 0.4; // 频率1
double freq2 = 0.7; // 频率2
double amp1 = 0.8; // 振幅1
double amp2 = 0.5; // 振幅2
draw_lissajous_curve(screen_width, screen_height, amp1, freq1, amp2, freq2);
return 0;
}
```
在这个示例中,我们首先导入必要的库,然后创建一个窗口并加载一个字体。接着,我们遍历屏幕上的每一个像素点,计算对应的两个正弦信号值,并使用它们作为颜色分量来填充每个像素。最后,我们在主循环中更新窗口显示。
阅读全文
相关推荐
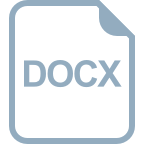
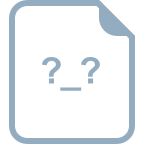

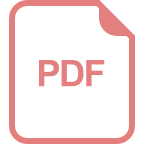
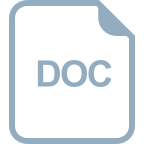
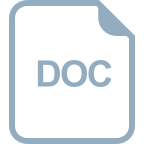






