mqtt上报结构体属性的消息
时间: 2023-08-28 13:03:38 浏览: 66
好的,针对您的问题,我可以给出以下示例代码:
假设我们定义了一个结构体:
```c
typedef struct {
int temperature;
int humidity;
char *location;
} SensorReading;
```
我们可以使用 MQTT 协议来上报该结构体的属性。
首先,我们需要定义一个 MQTT 主题,用于发送消息。例如,我们可以定义主题为 `sensors/1`。然后,我们可以将结构体的属性按照一定的格式打包成消息体,例如使用 JSON 格式:
```json
{
"temperature": 25,
"humidity": 60,
"location": "living room"
}
```
最后,我们将该消息体发送到 `sensors/1` 主题即可。
具体的代码实现可以参考下面的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <MQTTClient.h>
#include <cJSON.h>
#define ADDRESS "tcp://localhost:1883"
#define CLIENTID "ExampleClientPub"
#define TOPIC "sensors/1"
#define QOS 1
#define TIMEOUT 10000L
typedef struct {
int temperature;
int humidity;
char *location;
} SensorReading;
void send_reading(SensorReading *reading) {
MQTTClient client;
MQTTClient_connectOptions conn_opts = MQTTClient_connectOptions_initializer;
MQTTClient_message pubmsg = MQTTClient_message_initializer;
MQTTClient_deliveryToken token;
char *payload;
cJSON *root;
root = cJSON_CreateObject();
cJSON_AddNumberToObject(root, "temperature", reading->temperature);
cJSON_AddNumberToObject(root, "humidity", reading->humidity);
cJSON_AddStringToObject(root, "location", reading->location);
payload = cJSON_Print(root);
MQTTClient_create(&client, ADDRESS, CLIENTID, MQTTCLIENT_PERSISTENCE_NONE, NULL);
conn_opts.keepAliveInterval = 20;
conn_opts.cleansession = 1;
MQTTClient_connect(client, &conn_opts);
pubmsg.payload = payload;
pubmsg.payloadlen = strlen(payload);
pubmsg.qos = QOS;
pubmsg.retained = 0;
MQTTClient_publishMessage(client, TOPIC, &pubmsg, &token);
MQTTClient_waitForCompletion(client, token, TIMEOUT);
printf("Message with delivery token %d delivered\n", token);
MQTTClient_disconnect(client, 10000);
MQTTClient_destroy(&client);
cJSON_Delete(root);
free(payload);
}
int main(int argc, char* argv[]) {
SensorReading reading;
reading.temperature = 25;
reading.humidity = 60;
reading.location = "living room";
send_reading(&reading);
return EXIT_SUCCESS;
}
```
以上代码使用了 Eclipse Paho MQTT C 客户端库。首先,我们使用 cJSON 库将结构体打包成 JSON 格式的消息体,然后使用 MQTTClient_publishMessage 函数将消息发送到指定主题。在发送完成后,我们需要调用 MQTTClient_disconnect 函数关闭连接并释放资源。
希望这个示例能够帮到您,如果您还有其他问题,请继续提问。
相关推荐
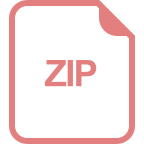
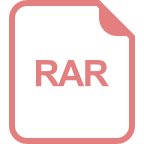














