完成项目:单页面组件实现与路由配置 要求:使用Vue.js实现网页内容,运用所学Vue有关的知识包括:数据绑定、内置指令、事件、方法、全局API、过渡动画、CLI、路由等 基本功能: 1、基于CLI环境开发 2、实现“home”组件。页面布局美观,结构清晰,有一定的业务逻辑与数据调用(可自由发挥) 3、实现“list”组件。页面布局美观,结构清晰,有一定的业务逻辑与数据调用(可自由发挥),有方法使用 4、实现“content”组件。页面布局美观,结构清晰 5、实现路由配置
时间: 2024-01-27 19:06:22 浏览: 19
很高兴接受这个项目,以下是一个简单的实现方案:
首先,我们需要安装 Vue CLI,可以在命令行中使用以下命令:
```
npm install -g @vue/cli
```
接着,创建一个新项目:
```
vue create my-project
```
在创建项目的时候,选择添加 Vue Router 插件,这样就可以自动配置好路由相关的文件和代码。
接下来,我们可以创建三个组件:Home、List 和 Content,分别对应网站的首页、列表页和内容页。这些组件可以放在 src/components 目录下。
在 Home 组件中,我们可以使用数据绑定来显示一些信息,例如:
```html
<template>
<div class="home">
<h1>{{ message }}</h1>
<p>{{ description }}</p>
<button @click="onClick">Click me</button>
</div>
</template>
<script>
export default {
data() {
return {
message: 'Welcome to my website',
description: 'This is a simple website built using Vue.js'
}
},
methods: {
onClick() {
alert('You clicked the button!')
}
}
}
</script>
<style>
/* Add some styles to make the page look nice */
</style>
```
在 List 组件中,我们可以使用 v-for 指令来循环显示一些数据,例如:
```html
<template>
<div class="list">
<h2>{{ title }}</h2>
<ul>
<li v-for="item in items" :key="item.id">
<a :href="'/content/' + item.id">{{ item.title }}</a>
</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
title: 'My blog posts',
items: [
{ id: 1, title: 'Post 1' },
{ id: 2, title: 'Post 2' },
{ id: 3, title: 'Post 3' }
]
}
}
}
</script>
<style>
/* Add some styles to make the page look nice */
</style>
```
在 Content 组件中,我们可以使用 props 来接收路由参数,并显示相应的内容,例如:
```html
<template>
<div class="content">
<h2>{{ title }}</h2>
<p>{{ content }}</p>
</div>
</template>
<script>
export default {
props: ['id'],
data() {
return {
title: '',
content: ''
}
},
created() {
// Load the content from the server using the ID
this.title = 'Post ' + this.id
this.content = 'This is the content of post ' + this.id
}
}
</script>
<style>
/* Add some styles to make the page look nice */
</style>
```
最后,我们需要在路由配置中将这些组件对应到不同的 URL 上,例如:
```javascript
import Vue from 'vue'
import VueRouter from 'vue-router'
import Home from '@/components/Home'
import List from '@/components/List'
import Content from '@/components/Content'
Vue.use(VueRouter)
const routes = [
{ path: '/', component: Home },
{ path: '/list', component: List },
{ path: '/content/:id', component: Content, props: true }
]
const router = new VueRouter({
routes
})
export default router
```
在这个配置中,/ 对应到 Home 组件,/list 对应到 List 组件,/content/:id 则对应到 Content 组件,并使用 props 来传递路由参数。
这样就完成了一个简单的使用 Vue.js 实现网页内容和路由配置的项目。当然,这只是一个示例,你可以根据自己的需求和想象来扩展和改进这个项目。
相关推荐
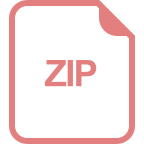
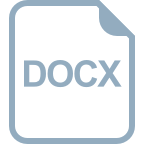
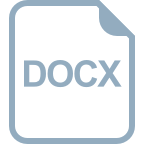








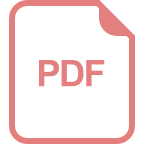
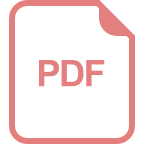
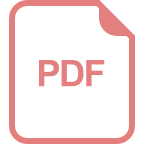
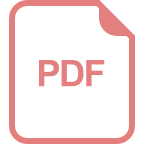
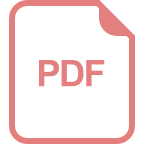
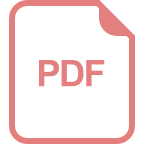
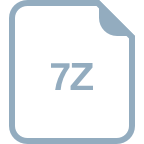