大型的Java游戏代码
时间: 2023-11-05 15:56:44 浏览: 45
大型的 Java 游戏代码是一个非常复杂的项目,需要使用多种技术和框架进行开发,包括游戏引擎、图形库、网络通信、数据库等。
以下是一个简单的 Java 游戏代码,使用了 JavaFX 图形库和多线程技术,实现了一个简单的打地鼠游戏:
```java
import javafx.animation.AnimationTimer;
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.GridPane;
import javafx.scene.layout.VBox;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.scene.text.Font;
import javafx.stage.Stage;
import java.util.Random;
public class WhackAMole extends Application {
private static final int ROWS = 3;
private static final int COLUMNS = 3;
private static final int MOLE_SIZE = 50;
private static final int PADDING = 10;
private Circle[][] holes;
private int score;
private Label scoreLabel;
@Override
public void start(Stage primaryStage) throws Exception {
BorderPane root = new BorderPane();
// 创建游戏区域
GridPane gridPane = new GridPane();
gridPane.setPadding(new Insets(PADDING));
gridPane.setHgap(PADDING);
gridPane.setVgap(PADDING);
holes = new Circle[ROWS][COLUMNS];
for (int row = 0; row < ROWS; row++) {
for (int col = 0; col < COLUMNS; col++) {
Circle hole = new Circle(MOLE_SIZE / 2);
hole.setFill(Color.GRAY);
hole.setStroke(Color.BLACK);
gridPane.add(hole, col, row);
holes[row][col] = hole;
}
}
root.setCenter(gridPane);
// 创建得分区域
VBox scoreBox = new VBox();
scoreBox.setAlignment(Pos.CENTER);
scoreLabel = new Label("得分:0");
scoreLabel.setFont(Font.font(18));
scoreBox.getChildren().add(scoreLabel);
root.setTop(scoreBox);
// 注册事件处理器
for (int row = 0; row < ROWS; row++) {
for (int col = 0; col < COLUMNS; col++) {
int finalRow = row;
int finalCol = col;
holes[row][col].setOnMouseClicked(event -> {
if (holes[finalRow][finalCol].getFill() == Color.RED) {
score++;
scoreLabel.setText("得分:" + score);
holes[finalRow][finalCol].setFill(Color.GRAY);
}
});
}
}
// 创建地鼠线程
Thread moleThread = new Thread(() -> {
Random random = new Random();
while (true) {
int row = random.nextInt(ROWS);
int col = random.nextInt(COLUMNS);
holes[row][col].setFill(Color.RED);
try {
Thread.sleep(random.nextInt(2000) + 1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
holes[row][col].setFill(Color.GRAY);
}
});
moleThread.setDaemon(true);
moleThread.start();
// 创建动画线程
AnimationTimer animationTimer = new AnimationTimer() {
@Override
public void handle(long now) {
for (int row = 0; row < ROWS; row++) {
for (int col = 0; col < COLUMNS; col++) {
if (holes[row][col].getFill() == Color.RED) {
holes[row][col].setRadius(MOLE_SIZE / 2 + MOLE_SIZE / 2 * Math.sin(now / 100_000_000.0));
}
}
}
}
};
animationTimer.start();
primaryStage.setScene(new Scene(root, MOLE_SIZE * COLUMNS + PADDING * (COLUMNS + 1), MOLE_SIZE * ROWS + PADDING * (ROWS + 1)));
primaryStage.setTitle("打地鼠游戏");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
```
这个游戏使用 JavaFX 图形库实现了一个 3x3 的地鼠打击游戏,玩家需要点击出现的红色圆圈来得分。游戏使用多线程技术实现了地鼠的随机出现,以及圆圈大小的动态变化效果。
相关推荐
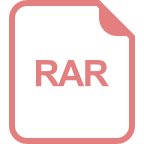
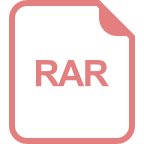














