我的意思是 用java写一个A*算法
时间: 2023-09-04 13:04:01 浏览: 134
### 回答1:
A* 算法是用来寻找最短路径的一种算法。其中,A* 算法使用了两个函数 f(n) 和 h(n)。函数 f(n) 表示从起点到达当前结点的真实距离,h(n) 表示从当前结点到目标结点的估算距离。A* 算法会不断地从开放列表中选取 f(n)+h(n) 最小的结点,并将其加入到封闭列表中。
要使用 Java 来实现 A* 算法,首先要定义一个结点类,包括结点的位置和父结点的位置,还有 f(n) 和 h(n) 函数的值。然后要定义一个类来实现 A* 算法,包括起点、终点、开放列表和封闭列表。在这个类中,你还需要实现对结点的操作,包括添加结点、删除结点和更新结点的信息。最后,你要实现 A* 算法的主要流程,包括找到当前结点的周围结点、更新结点信息以及判断是否已经找到了最短路径。
### 回答2:
A*算法是一种用于寻找最短路径的启发式搜索算法,常用于图形学、人工智能和游戏开发等领域。下面是用Java实现A*算法的示例代码:
```java
import java.util.*;
public class AStarAlgorithm {
private int[][] grid; // 存储地图信息
private int rows;
private int cols;
private Node startNode;
private Node endNode;
public AStarAlgorithm(int[][] grid, Node startNode, Node endNode) {
this.grid = grid;
this.rows = grid.length;
this.cols = grid[0].length;
this.startNode = startNode;
this.endNode = endNode;
}
public List<Node> findPath() {
List<Node> openList = new ArrayList<>(); // 存放待访问的节点
List<Node> closedList = new ArrayList<>(); // 存放已访问的节点
openList.add(startNode);
while (!openList.isEmpty()) {
Node currentNode = getLowestFCostNode(openList);
openList.remove(currentNode);
closedList.add(currentNode);
if (currentNode.equals(endNode)) {
return getPath(currentNode);
}
List<Node> neighbors = getNeighbors(currentNode);
for (Node neighbor : neighbors) {
if (closedList.contains(neighbor) || neighbor.isWall()) {
continue;
}
int gCost = currentNode.getGCost() + calculateDistance(currentNode, neighbor);
if (gCost < neighbor.getGCost() || !openList.contains(neighbor)) {
neighbor.setGCost(gCost);
neighbor.setHCost(calculateDistance(neighbor, endNode));
neighbor.setParent(currentNode);
if (!openList.contains(neighbor)) {
openList.add(neighbor);
}
}
}
}
return new ArrayList<>(); // 无法找到路径,返回空列表
}
private Node getLowestFCostNode(List<Node> nodeList) {
Node lowestFCostNode = nodeList.get(0);
for (Node node : nodeList) {
if (node.getFCost() < lowestFCostNode.getFCost()) {
lowestFCostNode = node;
}
}
return lowestFCostNode;
}
private List<Node> getNeighbors(Node node) {
List<Node> neighbors = new ArrayList<>();
int[] dx = {-1, 1, 0, 0};
int[] dy = {0, 0, -1, 1};
for (int i = 0; i < 4; i++) {
int newX = node.getX() + dx[i];
int newY = node.getY() + dy[i];
if (newX >= 0 && newX < rows && newY >= 0 && newY < cols) {
neighbors.add(new Node(newX, newY));
}
}
return neighbors;
}
private int calculateDistance(Node node1, Node node2) {
int dx = Math.abs(node1.getX() - node2.getX());
int dy = Math.abs(node1.getY() - node2.getY());
return dx + dy;
}
private List<Node> getPath(Node currentNode) {
List<Node> path = new ArrayList<>();
while (currentNode != null) {
path.add(0, currentNode);
currentNode = currentNode.getParent();
}
return path;
}
public static void main(String[] args) {
int[][] grid = {{0, 1, 0, 0, 0},
{0, 1, 0, 1, 0},
{0, 0, 0, 1, 0},
{0, 1, 1, 0, 0},
{0, 0, 0, 0, 0}};
Node startNode = new Node(0, 0);
Node endNode = new Node(4, 4);
AStarAlgorithm algorithm = new AStarAlgorithm(grid, startNode, endNode);
List<Node> path = algorithm.findPath();
for (Node node : path) {
System.out.println("(" + node.getX() + ", " + node.getY() + ")");
}
}
}
class Node {
private int x;
private int y;
private int gCost;
private int hCost;
private Node parent;
public Node(int x, int y) {
this.x = x;
this.y = y;
this.gCost = 0;
this.hCost = 0;
this.parent = null;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
public int getGCost() {
return gCost;
}
public void setGCost(int gCost) {
this.gCost = gCost;
}
public int getHCost() {
return hCost;
}
public void setHCost(int hCost) {
this.hCost = hCost;
}
public int getFCost() {
return gCost + hCost;
}
public boolean isWall() {
return grid[x][y] == 1;
}
public Node getParent() {
return parent;
}
public void setParent(Node parent) {
this.parent = parent;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (!(obj instanceof Node)) {
return false;
}
Node node = (Node) obj;
return x == node.x && y == node.y;
}
@Override
public int hashCode() {
return Objects.hash(x, y);
}
}
```
该代码实现了一个A*算法的类`AStarAlgorithm`,包括寻找最短路径的方法`findPath`、获取路径的方法`getPath`,以及辅助方法`getLowestFCostNode`、`getNeighbors`和`calculateDistance`。
在`main`方法中,我们定义了一个图形地图`grid`、起始节点`startNode`和目标节点`endNode`,然后使用A*算法找到最短路径,并将路径打印输出。
这个示例演示了如何使用Java编写A*算法来解决最短路径问题。
### 回答3:
A*算法是一种常用的路径搜索算法,可以用于找到起点到目标点的最短路径。在使用Java编写A*算法之前,我们需要了解一些基本概念和步骤。
首先,我们需要创建一个表示地图的数据结构。可以使用二维数组来表示,其中每个单元格代表地图上的一个节点。每个节点都有与其相邻的节点,我们可以通过数组索引来表示节点之间的连接。
然后,我们需要定义一些特殊节点,包括起点、目标点以及用于存储已访问和待访问节点的数据结构。可以使用优先队列(PriorityQueue)来存储待访问节点,并使用哈希表(HashMap)来存储已访问节点。
接下来,我们可以使用以下步骤来实现A*算法:
1. 将起点添加到待访问节点中,并将其路径成本设置为0。
2. 循环执行以下步骤,直到找到目标节点或待访问节点为空:
a. 从待访问节点中选择具有最小路径成本的节点,并将其标记为已访问。
b. 如果已访问的节点是目标节点,算法结束。
c. 否则,对于当前节点的每个相邻节点:
- 如果该节点已被访问过或是障碍物节点,则忽略它。
- 计算从起点到该节点的路径成本。
- 如果该节点已经在待访问节点中,并且新的路径成本更高,则忽略它。
- 否则,更新该节点的路径成本,并将其添加到待访问节点中。
3. 如果待访问节点为空,并且没有找到目标节点,则表示无法找到路径。
最后,我们可以根据已访问节点的路径信息,从目标节点向起点回溯,以获取最短路径。
以上是使用Java编写A*算法的一般步骤,具体实现需要根据具体的地图数据结构和算法细节进行调整。
阅读全文
相关推荐

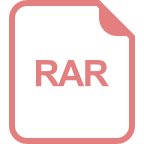












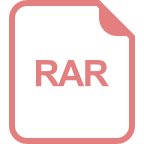
