java rsa oaep_Java加密算法 RSA
时间: 2024-01-04 12:03:47 浏览: 146
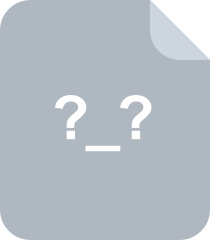
java实现的RSA加密算法

RSA算法是一种非对称加密算法,其中公钥可以用于加密数据,私钥则用于解密数据。而OAEP(Optimal Asymmetric Encryption Padding)是RSA算法加密时的一种填充方式,用于增加加密的安全性。在Java中,可以使用Java Cryptography Extension(JCE)提供的API来实现RSA算法和OAEP填充方式的加密与解密操作。以下是一个使用RSA算法和OAEP填充方式进行加密的Java示例代码:
```java
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import java.security.PublicKey;
import java.security.PrivateKey;
import javax.crypto.Cipher;
public class RSAEncryptor {
private static final String RSA_ALGORITHM = "RSA/ECB/OAEPWithSHA-256AndMGF1Padding";
public static void main(String[] args) throws Exception {
String plainText = "Hello, world!";
KeyPair keyPair = KeyPairGenerator.getInstance("RSA").generateKeyPair();
PublicKey publicKey = keyPair.getPublic();
PrivateKey privateKey = keyPair.getPrivate();
byte[] encryptedData = encrypt(plainText.getBytes(), publicKey);
byte[] decryptedData = decrypt(encryptedData, privateKey);
System.out.println("Plaintext: " + plainText);
System.out.println("Encrypted data: " + new String(encryptedData));
System.out.println("Decrypted data: " + new String(decryptedData));
}
public static byte[] encrypt(byte[] data, PublicKey publicKey) throws Exception {
Cipher cipher = Cipher.getInstance(RSA_ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
return cipher.doFinal(data);
}
public static byte[] decrypt(byte[] data, PrivateKey privateKey) throws Exception {
Cipher cipher = Cipher.getInstance(RSA_ALGORITHM);
cipher.init(Cipher.DECRYPT_MODE, privateKey);
return cipher.doFinal(data);
}
}
```
在这个示例代码中,我们使用KeyPairGenerator生成一个RSA密钥对,然后使用公钥加密一个字符串,最后使用私钥解密该字符串。注意,我们使用的RSA算法的填充方式为“OAEPWithSHA-256AndMGF1Padding”,这是一种较为安全的填充方式,可以提高加密的安全性。
阅读全文
相关推荐
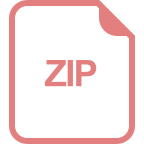
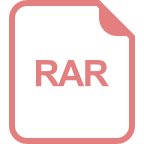
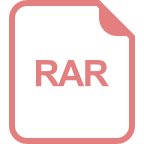
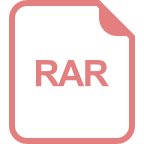
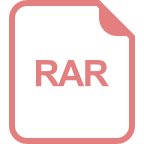
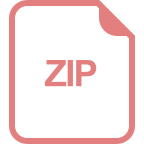
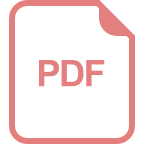
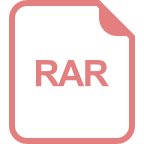
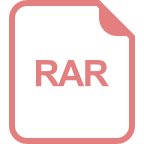
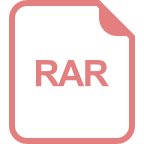
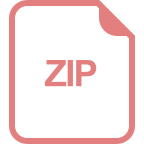
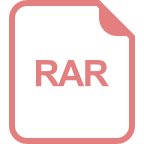


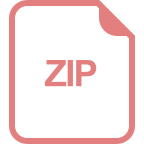
