读取文本文件将其插入数据库stu表中
时间: 2023-08-16 20:04:26 浏览: 53
好的,您可以使用以下 Python 代码实现:
```python
import sqlite3
# 连接到数据库
conn = sqlite3.connect('test.db')
c = conn.cursor()
# 创建 stu 表(如果不存在)
c.execute('''CREATE TABLE IF NOT EXISTS stu
(id INTEGER PRIMARY KEY AUTOINCREMENT,
name TEXT NOT NULL,
age INTEGER NOT NULL);''')
# 读取文本文件
with open('test.txt', 'r') as f:
lines = f.readlines()
# 将数据插入 stu 表
for line in lines:
name, age = line.strip().split(',')
c.execute("INSERT INTO stu (name, age) VALUES (?, ?)", (name, age))
# 提交更改并关闭连接
conn.commit()
conn.close()
```
其中,`test.db` 是数据库文件名,`stu` 表包含 `id`、`name` 和 `age` 三个字段,`test.txt` 是需要读取的文本文件。您需要将这些信息替换为您自己的文件名和字段名。
相关问题
将student表中的数计学院的学生信息插入到stu表中
### 回答1:
可以使用以下SQL语句将student表中数学院的学生信息插入到stu表中:
INSERT INTO stu (id, name, gender, age, major)
SELECT id, name, gender, age, major
FROM student
WHERE major = '数学院';
### 回答2:
为了将student表中数计学院的学生信息插入到stu表中,需要进行以下步骤:
1.首先,需要先建立一个新表stu,可以通过以下的SQL命令来创建:
CREATE TABLE stu(
id INT PRIMARY KEY,
name VARCHAR(20),
gender VARCHAR(4),
age INT,
department VARCHAR(50),
major VARCHAR(50)
);
2.然后,我们需要使用INSERT INTO语句来将student表中数计学院的学生信息插入到stu表中。INSERT INTO语句的语法如下:
INSERT INTO table_name (column1, column2, column3, ...)
VALUES (value1, value2, value3, ...);
具体到本次操作,我们可以使用以下SQL语句:
INSERT INTO stu (id, name, gender, age, department, major)
SELECT id, name, gender, age, department, major
FROM student
WHERE department = '数计学院';
此处,我们使用INSERT INTO语句将student表中符合‘数计学院’这一条件的学生信息插入到stu表中。其中,SELECT语句用于从student表中筛选符合条件的学生信息,然后将这些信息按照所在列的顺序插入到stu表中。
以上就是将student表中数计学院的学生信息插入到stu表中的步骤。在具体操作时,需注意SQL语句的语法正确以及数据类型等问题,以确保数据插入到新表中是准确无误的。
### 回答3:
首先需要确保student表和stu表已经存在,并且数计学院的学生信息已经在student表中全部添加完毕。
其次,需要使用SQL语句来将数计学院学生信息从student表中插入到stu表中。可以按照以下步骤操作:
1. 使用SELECT语句筛选出数计学院的学生信息,这里需要知道数计学院对应的院系编号或者名称。例如,如果该院系的编号为'001',则可以使用以下SQL语句:
SELECT * FROM student WHERE department_id='001';
2. 接着,可以将筛选出来的学生信息插入到stu表中。使用INSERT INTO语句来实现,具体语法如下:
INSERT INTO stu VALUES (学号, 姓名, 年龄, 性别, 院系编号, 班级);
这里需要注意,VALUES后面的值需要和stu表中的字段顺序一一对应,否则插入会失败。
3. 最后,需要执行以上SQL语句,将数计学院的学生信息插入到stu表中。在MySQL中,可以使用以下命令执行:
mysql> INSERT INTO stu VALUES (学号, 姓名, 年龄, 性别, 院系编号, 班级);
如果插入成功,会返回以下结果:
Query OK, 1 row affected
4. 最后再使用SELECT语句来验证插入结果是否正确。例如,在stu表中查找数计学院的学生信息,可以使用以下SQL语句:
SELECT * FROM stu WHERE department_id='001';
以上就是将student表中的数计学院的学生信息插入到stu表中的详细操作步骤。需要注意的是,在执行SQL语句时需要进行严格的字段匹配和数据格式验证,以免出现插入失败或者数据错乱等问题。
将student表中的数计学院的学生信息插入到stu表中。
可以使用如下SQL语句将student表中数学院的学生信息插入到stu表中:
INSERT INTO stu (id, name, age, gender, major)
SELECT id, name, age, gender, major
FROM student
WHERE major = '数学院';
相关推荐
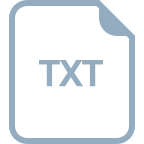
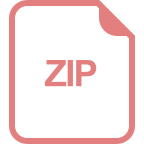
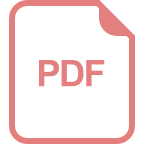









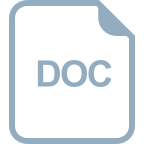
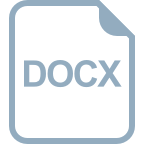
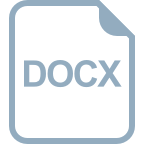
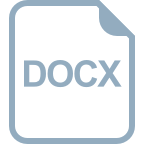