利用Scipy的integrate模块求解微分方程
时间: 2024-03-10 13:50:37 浏览: 17
好的,我可以回答这个问题。
Scipy 的 integrate 模块提供了多种方法来求解常微分方程(ODEs)和偏微分方程(PDEs),其中求解常微分方程的方法包括 odeint、ode、solve_ivp 等。
以求解一阶常微分方程为例,假设有如下微分方程:
y' = f(y, t)
其中 y 是未知函数,t 是自变量,f 是已知函数。可以使用 odeint 函数进行求解。首先需要导入 Scipy 和 Numpy 模块:
```python
import numpy as np
from scipy.integrate import odeint
```
然后定义微分方程的函数,例如:
```python
def f(y, t):
return -2 * y + np.sin(2 * t)
```
其中, y 是未知函数, t 是自变量。这个例子中,微分方程是 y' = -2y + sin(2t)。
然后定义初值条件:
```python
y0 = 0.5
```
最后调用 odeint 函数进行求解:
```python
t = np.linspace(0, 10, 101)
sol = odeint(f, y0, t)
```
这里将求解结果存储在 sol 变量中,t 是时间向量。
希望这个回答能够帮助你解决问题。
相关问题
python求解微分方程
在 Python 中,可以使用 SciPy 库来求解微分方程。SciPy 提供了一个专门的模块,称为 `scipy.integrate`,其中包含了一些函数可以用来求解常微分方程(ODEs)。
首先,你需要导入 `scipy.integrate` 模块中的 `odeint` 函数。然后,定义你的微分方程函数,该函数接受当前的状态和时间作为输入,并返回状态的导数。
以下是一个简单的示例,解决了一个简单的一阶微分方程:
```python
import numpy as np
from scipy.integrate import odeint
# 定义微分方程函数
def dydt(y, t):
return -2 * y # 示例微分方程为 dy/dt = -2y
# 定义初始条件
y0 = 1 # y 的初始值
t = np.linspace(0, 5, 100) # 时间范围
# 求解微分方程
y = odeint(dydt, y0, t)
# 打印结果
print(y)
```
这段代码将打印出在给定时间范围内的微分方程的解。你可以根据自己的微分方程进行修改。
希望这能帮到你!如果有任何问题,请随时问我。
python 求解微分方程组
在Python中,可以使用SciPy库来求解微分方程组。SciPy库中的`odeint`函数可以用于求解常微分方程组。
首先,需要导入必要的库:
```python
import numpy as np
from scipy.integrate import odeint
```
然后,定义微分方程组的函数。例如,假设我们要求解以下的微分方程组:
```
dy1/dt = f1(y1, y2, t)
dy2/dt = f2(y1, y2, t)
```
可以定义一个函数来表示这个微分方程组:
```python
def model(y, t):
y1, y2 = y
dy1dt = f1(y1, y2, t)
dy2dt = f2(y1, y2, t)
return [dy1dt, dy2dt]
```
其中,`y`是一个包含变量`y1`和`y2`的列表,`t`是时间。
接下来,需要定义函数`f1`和`f2`,它们表示微分方程组中的具体函数形式。
最后,需要指定初始条件和时间范围,并调用`odeint`函数进行求解:
```python
y0 = [y1_0, y2_0] # 初始条件
t = np.linspace(t_start, t_end, num_points) # 时间范围
sol = odeint(model, y0, t) # 求解微分方程组
```
其中,`sol`是一个包含求解结果的数组。
请注意,以上只是一个简单的示例,实际的微分方程组可能更加复杂。你需要根据具体的微分方程组来定义相应的函数和初始条件。
相关推荐
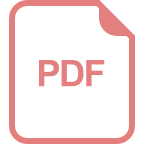












