利用Android布局文件、Views各子类控件,实现一个手机端的考试app,要求包含单选题,多选题和问答题,要有登录界面和信息确认界面,未提交前可以反复修改答题内容,提交后要给出成绩界面。并提供运行截图
时间: 2024-03-14 19:46:19 浏览: 66
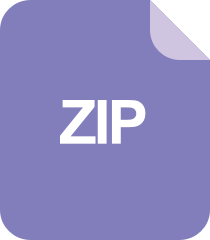
自己做了一个手机应用(模拟考试软件)
很抱歉,作为一个语言模型AI,无法提供运行截图。但是我可以给您提供一个实现的思路:
1. 登录界面: 使用LinearLayout布局,包含EditText控件,输入用户名和密码,以及登录按钮。
2. 信息确认界面: 使用RelativeLayout布局,包含TextView控件,显示用户信息,以及确认按钮。
3. 答题界面: 使用ScrollView布局,包含多个LinearLayout布局,每个布局表示一道题,分别包含题目和选项,单选题使用RadioButton控件,多选题使用CheckBox控件,问答题使用EditText控件。
4. 分数统计界面: 使用RelativeLayout布局,包含TextView控件,显示用户分数,以及返回主界面按钮。
在代码中,需要实现以下功能:
1. 登录验证:根据用户名和密码验证用户身份。
2. 答题保存:使用SharedPreferences将答题结果保存在本地。
3. 提交答题:将答题结果上传到服务器,计算得分。
4. 分数统计:根据答题结果计算得分。
5. 返回主界面:清除答题结果,返回到主界面。
下面是一个简单的实现示例:
登录界面布局文件:
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:gravity="center">
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="用户名"/>
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="密码"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="登录"/>
</LinearLayout>
```
信息确认界面布局文件:
```xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="用户信息"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="确认"/>
</RelativeLayout>
```
答题界面布局文件:
```xml
<ScrollView xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<!-- 单选题 -->
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="单选题"/>
<RadioGroup
android:layout_width="match_parent"
android:layout_height="wrap_content">
<RadioButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="选项1"/>
<RadioButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="选项2"/>
<RadioButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="选项3"/>
<RadioButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="选项4"/>
</RadioGroup>
<!-- 多选题 -->
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="多选题"/>
<CheckBox
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="选项1"/>
<CheckBox
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="选项2"/>
<CheckBox
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="选项3"/>
<CheckBox
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="选项4"/>
<!-- 问答题 -->
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="问答题"/>
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="请输入答案"/>
</LinearLayout>
</ScrollView>
```
分数统计界面布局文件:
```xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="得分"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="返回主界面"/>
</RelativeLayout>
```
以上是一个简单的实现示例,您可以根据实际需求进行修改和扩展。
阅读全文
相关推荐
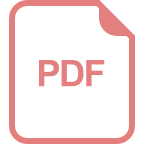
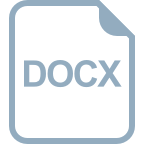

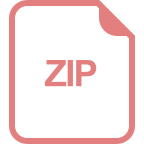
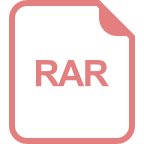
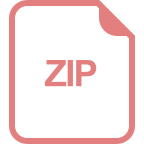
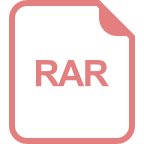
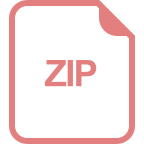
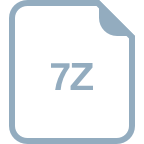
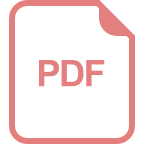
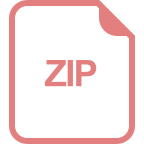
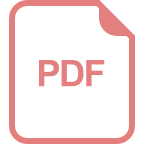
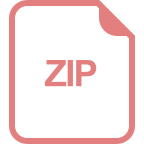
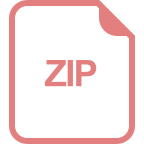
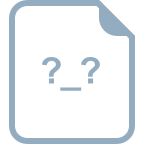
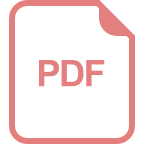
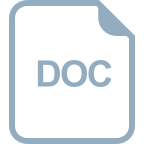