onceperrequestfilter里清除threadlocal
时间: 2023-08-14 10:08:53 浏览: 98
### 回答1:
在 Django 中,使用 `ThreadLocal` 变量可以在每个请求处理期间将变量存储在线程本地存储中。为了确保在每个请求中使用干净的线程本地存储,可以在 `onceperrequestfilter` 中清除 `ThreadLocal` 变量。
以下是一个示例代码:
```python
import threading
_thread_locals = threading.local()
def get_current_request():
return getattr(_thread_locals, 'request', None)
class OncePerRequestMiddleware:
def __init__(self, get_response):
self.get_response = get_response
def __call__(self, request):
_thread_locals.request = request
response = self.get_response(request)
return response
def process_response(self, request, response):
# 清除 ThreadLocal 变量,以确保它们不会泄漏到下一个请求中
_thread_locals.request = None
return response
```
在这个中间件中,我们将 `request` 对象存储在 `ThreadLocal` 变量 `_thread_locals` 中。在每个请求处理期间,我们可以使用 `get_current_request()` 函数来获取当前请求的 `request` 对象。在 `process_response()` 方法中,我们清除了 `_thread_locals` 变量,以确保它们不会泄漏到下一个请求中。
### 回答2:
在实现OncePerRequestFilter类的过滤器中,清除ThreadLocal需要遵循以下步骤:
1. 声明一个ThreadLocal变量,例如:private static ThreadLocal<CustomObject> threadLocal = new ThreadLocal<>();
2. 重写OncePerRequestFilter类中的doFilterInternal方法,在方法的开始位置获取当前线程的ThreadLocal对象的值,并将其存储在一个临时变量中,以便稍后使用。
3. 在doFilterInternal方法的try-catch-finally块中进行过滤操作。
4. 在结束过滤操作之后,无论是否发生异常,都需要使用finally块来清除ThreadLocal变量。
下面是一个示例代码片段来说明如何在OncePerRequestFilter类中清除ThreadLocal:
```java
public class CustomFilter extends OncePerRequestFilter {
private static ThreadLocal<CustomObject> threadLocal = new ThreadLocal<>();
@Override
protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain filterChain) throws ServletException, IOException {
CustomObject customObject = threadLocal.get();
try {
// 进行过滤操作
filterChain.doFilter(request, response);
} finally {
threadLocal.remove(); // 清除ThreadLocal变量
}
}
}
```
上述代码片段演示了如何在doFilterInternal方法中清除ThreadLocal变量。在try-catch-finally块中,过滤操作将在调用filterChain.doFilter方法之前和之后执行。在finally块中,我们使用threadLocal.remove()方法显式地清除ThreadLocal变量,以确保在线程结束时正确清除ThreadLocal值。
通过这种方式,我们可以在使用OncePerRequestFilter过滤器时清除ThreadLocal变量,以防止数据污染和内存泄漏问题。
阅读全文
相关推荐
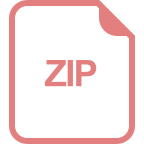
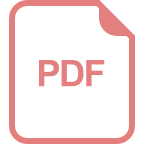
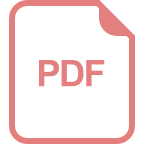















