编写一个函数求字符串长度,函数名为stringlen
时间: 2024-05-16 10:14:09 浏览: 24
以下是用C语言编写的函数stringlen,可以求出输入字符串的长度:
```c
#include <stdio.h>
int stringlen(char* str) {
int len = 0;
while (*str++ != '\0') {
len++;
}
return len;
}
int main() {
char str[] = "Hello World!";
int len = stringlen(str);
printf("The length of the string is %d\n", len);
return 0;
}
```
函数中使用了一个while循环,遍历字符串中每个字符,直到遇到字符串结束符'\0'为止。每遍历一个字符,计数器len加1。最后返回计数器的值,即为字符串的长度。在main函数中调用stringlen函数,并输出结果。
相关问题
汇编 在目的字符串插入另一个字符串
下面是一个基本的汇编程序,将一个字符串插入到另一个字符串中:
```assembly
section .data
string db 'Hello, world!',0
insert db ' there',0
section .text
global _start
_start:
mov ecx,0 ; counter for length of string
mov ebx,string ; pointer to string
mov eax,insert ; pointer to insert string
mov edi,ebx ; destination pointer to string
repne scasb ; find null terminator in string
dec edi ; move back one byte to point to null terminator
mov esi,eax ; source pointer to insert string
mov ecx,insertLen ; length of insert string
rep movsb ; copy insert string to destination
mov ecx,stringLen+insertLen ; total length of new string
mov eax,4 ; system call for write
mov ebx,1 ; file descriptor for stdout
mov edx,ecx ; length of new string
mov ecx,string ; pointer to new string
int 0x80 ; call kernel
mov eax,1 ; system call for exit
xor ebx,ebx ; exit code 0
int 0x80 ; call kernel
section .bss
stringLen resb 1 ; length of string
insertLen resb 1 ; length of insert string
```
在上面的程序中,我们首先定义了两个字符串:`string`和`insert`。然后,在程序中,我们使用了一些汇编指令来将一个字符串插入到另一个字符串中。以下是程序的工作原理:
1. 首先,我们使用`mov`指令将两个字符串的地址分别存储在`ebx`和`eax`寄存器中。
2. 然后,我们使用`repne scasb`指令来查找第一个字符串中的空字符(`0`)。
3. 接下来,我们将`edi`寄存器中的值减去`1`,以便它指向第一个字符串的末尾。
4. 然后,我们将`esi`寄存器中的值设置为第二个字符串的起始地址,将`ecx`寄存器中的值设置为第二个字符串的长度,并使用`rep movsb`指令将第二个字符串复制到第一个字符串中。
5. 最后,我们使用`mov`指令将`eax`、`ebx`和`ecx`寄存器分别设置为`4`、`1`和新字符串的长度,并使用`int 0x80`指令调用内核来输出新字符串。然后,我们使用`mov`指令将`eax`和`ebx`寄存器分别设置为`1`和`0`,并使用`int 0x80`指令调用内核来退出程序。
这里需要注意的是,在程序的末尾,我们使用了`resb`指令来定义了两个变量`stringLen`和`insertLen`。这些变量用于存储第一个字符串和第二个字符串的长度,因为汇编中没有内置的字符串长度函数。
用重载函数实现一组数的升序排序,分别考虑整数,单精度浮点数,字符型数据,字符串数据
### 回答1:
好的,我可以回答这个问题。以下是用重载函数实现一组数的升序排序的代码示例:
```
#include <iostream>
#include <string>
using namespace std;
void sort(int arr[], int n) {
for (int i = ; i < n - 1; i++) {
for (int j = ; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
void sort(float arr[], int n) {
for (int i = ; i < n - 1; i++) {
for (int j = ; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
float temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
void sort(char arr[], int n) {
for (int i = ; i < n - 1; i++) {
for (int j = ; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
char temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
void sort(string arr[], int n) {
for (int i = ; i < n - 1; i++) {
for (int j = ; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
string temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
int main() {
int intArr[] = { 3, 1, 4, 1, 5, 9, 2, 6, 5, 3 };
float floatArr[] = { 3.14, 1.41, 2.72, 1.61, .62 };
char charArr[] = { 'c', 'a', 't', 'd', 'o', 'g' };
string stringArr[] = { "apple", "banana", "orange", "pear", "grape" };
int intLen = sizeof(intArr) / sizeof(int);
int floatLen = sizeof(floatArr) / sizeof(float);
int charLen = sizeof(charArr) / sizeof(char);
int stringLen = sizeof(stringArr) / sizeof(string);
sort(intArr, intLen);
sort(floatArr, floatLen);
sort(charArr, charLen);
sort(stringArr, stringLen);
cout << "Sorted int array: ";
for (int i = ; i < intLen; i++) {
cout << intArr[i] << " ";
}
cout << endl;
cout << "Sorted float array: ";
for (int i = ; i < floatLen; i++) {
cout << floatArr[i] << " ";
}
cout << endl;
cout << "Sorted char array: ";
for (int i = ; i < charLen; i++) {
cout << charArr[i] << " ";
}
cout << endl;
cout << "Sorted string array: ";
for (int i = ; i < stringLen; i++) {
cout << stringArr[i] << " ";
}
cout << endl;
return ;
}
```
希望这个代码示例能够帮到你!
### 回答2:
重载函数是指在同一个类中定义多个同名函数,但它们的参数类型或参数个数不同。
要实现一组数的升序排序,我们可以使用重载函数来处理不同类型的数据。我们可以定义一个含有一个参数的函数sort,参数类型为整数数组,该函数可以对整数数组进行升序排序。同样地,我们可以定义相同名字的函数sort,但参数类型为单精度浮点数数组,字符型数组和字符串数组。
对于整数数组的排序函数,我们可以使用著名的排序算法,如冒泡排序或快速排序来实现。排序后,我们返回升序排序后的整数数组。
对于单精度浮点数数组的排序函数,我们可以使用相同的排序算法来实现,只需稍微修改条件和比较操作符。排序后,我们返回升序排序后的单精度浮点数数组。
对于字符型数组的排序函数,我们可以使用类似整数数组的排序算法,但改为按照字符的ASCII值进行比较和交换操作。排序后,我们返回升序排序后的字符型数组。
对于字符串数组的排序函数,我们可以使用类似单精度浮点数数组的排序算法,但改为按照字符串的字典顺序进行比较和交换操作。排序后,我们返回升序排序后的字符串数组。
通过使用重载函数,我们可以根据不同的数据类型来调用适当的排序函数,使得排序过程更加灵活和简便。这种方法可以增加程序的可读性和可维护性,同时也提高了代码的重用性。
### 回答3:
可以通过重载函数实现一组数的升序排序。我们可以分别考虑整数、单精度浮点数、字符型数据和字符串数据的情况。具体实现如下:
1. 对于整数的排序,可以定义一个函数`sort`,函数参数为一个整数数组和数组的长度。在函数内部,可以使用常见的排序算法(如冒泡排序或快速排序)对整数数组进行升序排序。
2. 对于单精度浮点数的排序,同样定义一个函数`sort`,函数参数为一个单精度浮点数数组和数组的长度。在函数内部,同样使用排序算法对单精度浮点数数组进行升序排序。
3. 对于字符型数据的排序,同样定义一个函数`sort`,函数参数为一个字符型数组和数组的长度。在函数内部,同样使用排序算法对字符型数组进行升序排序。可以使用`strcmp`函数比较字符的大小进行排序。
4. 对于字符串数据的排序,同样定义一个函数`sort`,函数参数为一个字符串数组和数组的长度。在函数内部,同样使用排序算法对字符串数组进行升序排序。可以使用`strcmp`函数比较字符串的大小进行排序。
通过重载函数的方式,我们可以根据参数类型的不同,调用对应的排序函数,从而实现一组数的升序排序。这样的实现不仅提高了代码的复用性,还可以更好地满足不同类型数据的排序需求。
相关推荐
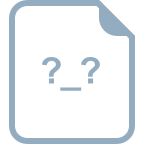






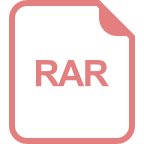
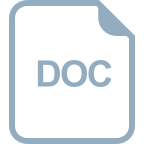
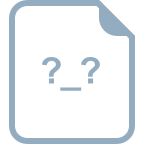
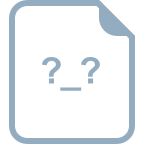
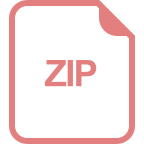