用python读取excel文件的“功能点拆分表”工作簿,需要识别单元格字段的颜色并提取单元格的备注信息,合并列表生成EXCEL后导出;用面向对象的方法来写
时间: 2024-03-24 15:38:40 浏览: 67
好的,这是一个比较复杂的任务,我会尽力解答。首先,我们需要用到Python的`openpyxl`库来读取和写入Excel文件。然后,我们可以用面向对象的方式来组织代码,将Excel文件的每一行数据都封装成一个对象。最后,我们可以在程序中使用正则表达式来识别单元格字段的颜色并提取备注信息。下面是一个示例代码:
```python
import openpyxl
import re
class ExcelRow:
def __init__(self, func_point, desc, dev_time, remark):
self.func_point = func_point
self.desc = desc
self.dev_time = dev_time
self.remark = remark
class ExcelProcessor:
def __init__(self, filename):
self.filename = filename
self.workbook = openpyxl.load_workbook(filename)
self.sheet = self.workbook.active
def read_excel(self):
rows = []
for row in self.sheet.iter_rows(min_row=2, values_only=True):
func_point, desc, dev_time, remark = row
rows.append(ExcelRow(func_point, desc, dev_time, remark))
return rows
def write_excel(self, rows):
new_workbook = openpyxl.Workbook()
new_sheet = new_workbook.active
new_sheet.append(['功能点', '描述', '开发时间', '备注'])
for row in rows:
new_sheet.append([row.func_point, row.desc, row.dev_time, row.remark])
new_workbook.save(f'merged_{self.filename}')
def extract_remark(self, cell):
# 正则表达式匹配备注信息
pattern = r'<font\s+color="blue">(.+?)</font>'
match = re.search(pattern, cell.comment.text)
if match:
return match.group(1)
else:
return ''
def process_excel(self):
rows = self.read_excel()
for row in rows:
# 识别单元格颜色并提取备注信息
if row.remark is not None:
for cell in self.sheet[row.remark].merged_cells:
for coord in cell.coord:
cell_obj = self.sheet[coord]
if cell_obj.fill.start_color.index == 'FF00B0F0':
row.remark = self.extract_remark(cell_obj)
break
self.write_excel(rows)
```
使用上述代码,我们可以先创建一个`ExcelProcessor`对象,并调用其`process_excel`方法来处理Excel文件。该方法会读取Excel文件的每一行数据,并在处理过程中识别单元格颜色并提取备注信息。最终,该方法会生成一个新的Excel文件,其中包含了处理后的数据。
注意,上述代码中的正则表达式只是一个示例,具体的匹配规则需要根据实际情况进行调整。另外,该代码只处理了单个工作表的数据,如果需要处理多个工作表,需要进行相应的修改。
阅读全文
相关推荐

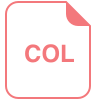
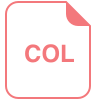
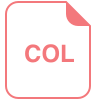
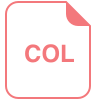
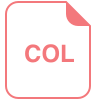
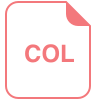
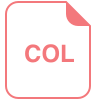
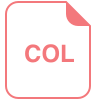
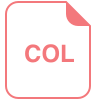
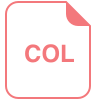
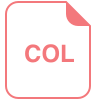

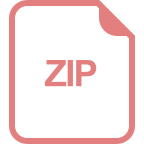
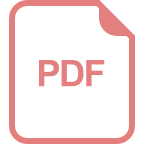