python怎么判断文件是什么编码?
时间: 2024-05-12 19:15:11 浏览: 13
Python可以使用第三方库`chardet`来判断文件的编码格式,它可以自动识别多种编码格式。
安装`chardet`库:
```
pip install chardet
```
判断文件编码的示例代码:
```python
import chardet
# 读取文件二进制数据
with open('file.txt', 'rb') as f:
data = f.read()
# 利用chardet库检测编码格式
result = chardet.detect(data)
print(result['encoding'])
```
`chardet.detect()`函数返回一个字典,其中`encoding`键对应的值表示检测到的编码格式。如果返回值为`None`,则表示无法识别编码格式。
相关问题
python判断文件编码类型
Python 库 chardet 可以判断文件编码类型。你可以使用如下代码:
```python
import chardet
with open('file.txt', 'rb') as f:
result = chardet.detect(f.read())
print(result['encoding'])
```
其中,参数 `'file.txt'` 需要替换成你想要判断编码类型的文件路径。`chardet.detect()` 函数返回一个字典,其中 `'encoding'` 键对应该文件的编码类型。
Python解释器默认编码是什么?如何修改?
Python解释器默认编码是UTF-8。你可以通过在Python脚本的第一行添加以下注释来修改默认编码:
```python
# -*- coding: 编码 -*-
```
其中,"编码"可以替换为你想要设置的编码,例如:
```python
# -*- coding: utf-8 -*-
```
这样设置后,Python解释器会使用指定的编码来读取源代码文件。请注意,这只会影响源代码文件的编码,对于使用Unicode字符串和Unicode字符的程序可能仍然需要进行额外的编码和解码操作。
相关推荐
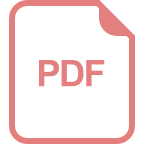
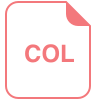
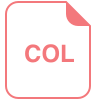
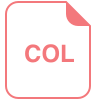
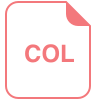
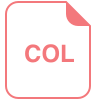







