python自定义函数,实现一个简单的累加器
时间: 2024-04-30 08:25:12 浏览: 23
可以通过以下代码实现一个简单的累加器:
```python
def accumulator():
total = 0
def add(x):
nonlocal total
total += x
return total
return add
```
然后我们可以使用这个函数来创建一个累加器实例:
```python
acc = accumulator()
```
接着就可以通过调用 `acc` 函数来不断累加数值了:
```python
print(acc(1)) # 输出 1
print(acc(2)) # 输出 3
print(acc(3)) # 输出 6
```
在这个实现中,我们使用了闭包和 `nonlocal` 关键字来实现了一个可以记住累加结果的函数。
相关问题
python自定义函数累加器
Python中的自定义函数累加器可以通过定义一个变量,在每次函数被调用时对该变量进行更新来实现。以下是一个简单的示例:
```python
def accumulator():
total = 0
def add(value):
nonlocal total
total += value
return total
return add
```
这个累加器函数定义了一个内部函数`add`,它有一个参数`value`,每次调用`add`时会将该参数加到一个名为`total`的变量中,然后返回当前`total`的值。注意,使用`nonlocal`关键字来声明`total`变量,这样在内部函数中就可以修改外部函数的变量了。
使用示例:
```python
acc = accumulator()
print(acc(1)) # 1
print(acc(2)) # 3
print(acc(3)) # 6
```
以上代码中,首先调用`accumulator()`函数得到一个`add`函数的引用,然后通过不断调用`add`函数来累加数值。
自定义函数,实现一个简单地累加器
好的,我可以帮你写一个简单的累加器函数。以下是代码示例:
```python
def accumulator():
total = 0
while True:
x = yield total
total += x
```
这个函数使用了 Python 的生成器(generator)来实现。它会持续地接受输入的数值并将其累加,每次累加后会返回当前的总和。你可以这样使用它:
```python
acc = accumulator()
next(acc) # 必须先调用一次 next(),让生成器准备好接受输入
print(acc.send(1)) # 输出 1,当前总和为 1
print(acc.send(2)) # 输出 3,当前总和为 1+2=3
print(acc.send(3)) # 输出 6,当前总和为 1+2+3=6
```
希望这个累加器函数能够满足你的需求!
相关推荐
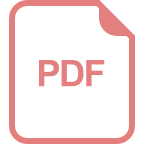
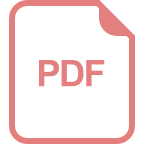
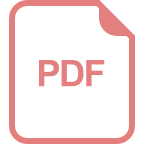
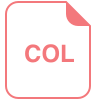
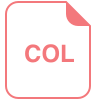
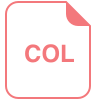
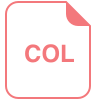
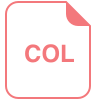






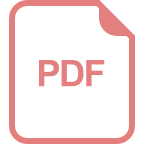
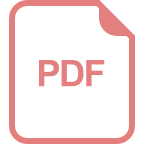