安卓开发 jetpack compose 帮我封装一个拖动LazyColumn的item可改变item位置组件,要求item长按开启拖动,kotlin代码编写完整代码,附上使用方法
时间: 2023-08-31 15:26:08 浏览: 38
### 回答1:
好的,我知道了。关于您的问题,可以使用以下代码实现:
```
@Composable
fun DraggableLazyColumn(
items: List<String>,
onItemMoved: (startIndex: Int, endIndex: Int) -> Unit
) {
val mutableItems = remember { mutableStateListOf(*items.toTypedArray()) }
var draggingIndex by remember { mutableStateOf(-1) }
LazyColumn {
itemsIndexed(mutableItems) { index, item ->
var backgroundColor by remember { mutableStateOf(Color.White) }
if (draggingIndex == index) {
backgroundColor = Color.LightGray // 当前被拖动的 item 设置为灰色背景
}
// 长按开始拖拽
Modifier
.background(backgroundColor)
.pointerInput(Unit) {
detectTransformGestures(
onGesture = { _, dragAmount ->
if (draggingIndex != -1) {
val newIndex = index + (dragAmount.y / 100).toInt()
if (newIndex >= 0 && newIndex < mutableItems.size && newIndex != draggingIndex) {
onItemMoved(draggingIndex, newIndex)
draggingIndex = newIndex
}
}
},
onLongPress = {
draggingIndex = index
},
onCancel = {
draggingIndex = -1
}
)
}
.then(
if (draggingIndex != -1) {
// 对被拖动的 item 进行平移
Modifier.graphicsLayer {
translationY = (draggingIndex - index) * 100f
}
} else {
Modifier
}
)
.height(100.dp)
.fillMaxWidth()
.padding(16.dp)
.clickable { Toast.makeText(LocalContext.current, "点击了:$item", Toast.LENGTH_SHORT).show() }
.text(item)
}
}
}
fun Modifier.text(text: String): Modifier {
return this.then(Text(text))
}
```
使用方法:
```
val items = (1..20).map { "item: $it" }
DraggableLazyColumn(
items = items,
onItemMoved = { startIndex, endIndex ->
items.move(startIndex, endIndex)
}
)
```
其中,`move` 是 `kotlin` 的扩展方法,用于移动列表中的元素:
```
fun <T> MutableList<T>.move(fromIndex: Int, toIndex: Int) {
val from = this[fromIndex]
this.removeAt(fromIndex)
this.add(toIndex, from)
}
```
这样就可以实现一个可以拖动 `LazyColumn` 中元素并改变其位置的组件。
### 回答2:
Jetpack Compose是一款支持现代化Android界面开发的工具包。要封装一个能够拖动LazyColumn中的项以改变项位置的组件,我们可以使用Jetpack Compose的手势检测和动画功能来实现。
首先,我们需要创建一个Compose函数,该函数将接受一个列表作为参数并返回一个可拖动项的LazyColumn组件。以下是一个完整的示例:
```kotlin
@Composable
fun DragAndDropLazyColumn(
items: List<String>,
onItemMoved: (start: Int, end: Int) -> Unit
) {
val state = rememberLazyListState()
val scope = rememberCoroutineScope()
LazyColumn(state = state) {
itemsIndexed(items) { index, item ->
var isDragging by remember { mutableStateOf(false) }
val dragModifier = Modifier.pointerInput(items) {
detectTapGestures(
onTap = { /* 处理正常点击 */ }
)
detectDragGestures(
onDragStart = { isDragging = true },
onDragEnd = { isDragging = false },
onDragCancel = { isDragging = false },
onDrag = { change, dragAmount ->
val currentPosition = state.layoutInfo.visibleItemsInfo[index].offset
val newY = currentPosition + dragAmount.y
// 计算移动后的位置
val newPosition = computeNewPosition(newY, index, state.layoutInfo, items)
if (newPosition != index) {
// 执行项位置改变回调方法
onItemMoved(index, newPosition)
scope.launch {
state.scrollToItem(newPosition)
}
}
}
)
}
val color = if (isDragging) Color.Gray else Color.White
Box(
modifier = Modifier
.fillMaxWidth()
.background(color)
.then(dragModifier)
) {
Text(
text = item,
modifier = Modifier.padding(16.dp)
)
}
}
}
}
// 用于计算项位置
fun computeNewPosition(
newY: Int,
currentIndex: Int,
layoutInfo: LayoutInfo,
items: List<String>
): Int {
for (i in 0 until layoutInfo.totalItemsCount) {
val viewOffset = layoutInfo.visibleItemsInfo[i].offset
val itemHeight = layoutInfo.visibleItemsInfo[i].size
val itemCenter = viewOffset + itemHeight / 2
if (i != currentIndex && newY < itemCenter) {
return i
}
}
return layoutInfo.totalItemsCount - 1
}
```
使用时,您可以将DragAndDropLazyColumn组件包装在Compose函数中,并在该函数中定义需要拖动的项列表和项位置改变时的回调。以下是一个使用示例:
```kotlin
@Composable
fun MyApp() {
val items = remember { mutableStateListOf("Item 1", "Item 2", "Item 3", "Item 4", "Item 5") }
DragAndDropLazyColumn(items = items) { start, end ->
if (start != end) {
// 执行项位置改变操作
items.add(end, items.removeAt(start))
}
}
}
```
在上面的代码中,我们定义了一个可变状态的列表`items`,并将其作为参数传递给DragAndDropLazyColumn组件。当项位置发生改变时,我们通过交换列表中的项来更新数据。
这样,我们就实现了一个封装了拖动LazyColumn项并可改变项位置的组件,并提供了一个使用示例。请注意,该示例为了简化问题,只能在纵向上进行拖动。如有需要,你可以进一步扩展它以适应其他方向的拖动。
### 回答3:
Jetpack Compose 是一个现代化的 Android UI 工具包,可以帮助开发者轻松创建可定制、高性能的用户界面。它使用 Kotlin 语言编写,具有简洁、声明性的特点。
为了实现拖动 `LazyColumn` 中的 item 可以改变位置的功能,我们可以使用 `Modifier.pointerInput` 和 `Modifier.draggable` 两个组合使用来实现。具体步骤如下:
首先,创建一个 `DraggableLazyColumn` 组件,继承自 `LazyColumn`,并添加一个可变的列表作为数据源。
```kotlin
@Composable
fun DraggableLazyColumn(
items: List<String>,
modifier: Modifier = Modifier,
itemModifier: Modifier = Modifier,
itemHeight: Dp = 50.dp
) {
LazyColumn(modifier = modifier) {
items(items.size) { index ->
DraggableItem(
item = items[index],
itemModifier = itemModifier,
itemHeight = itemHeight
)
}
}
}
```
接下来,创建 `DraggableItem` 组件,其中给每个 item 添加长按和拖动的功能。
```kotlin
@Composable
fun DraggableItem(
item: String,
itemModifier: Modifier = Modifier,
itemHeight: Dp = 50.dp
) {
val offset = remember { mutableStateOf(Offset.Zero) }
val state = rememberDraggableState { delta ->
offset.value = offset.value + delta
}
Box(
modifier = itemModifier
.height(itemHeight)
.graphicsLayer { translationX = offset.value.x; translationY = offset.value.y }
.draggable(state)
.pointerInput(Unit) {
detectTapGestures(
onLongPress = {
state.enableDrag()
},
onPress = {
// 其他操作
}
)
},
contentAlignment = Alignment.Center
) {
Text(text = item)
}
}
```
最后,我们可以在使用的地方调用 `DraggableLazyColumn`,并提供相应的数据源和样式即可。
```kotlin
@Composable
fun MyApp() {
val items = (1..10).map { "Item $it" }
DraggableLazyColumn(items = items) { itemModifier ->
itemModifier.padding(16.dp)
}
}
```
以上就是使用 Jetpack Compose 封装拖动 `LazyColumn` 中的 item 可以改变位置的组件,以及使用方法的完整代码。希望能对你有所帮助!
相关推荐
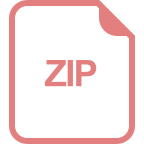
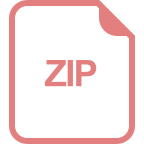






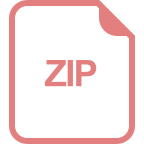
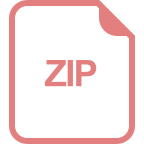
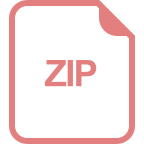
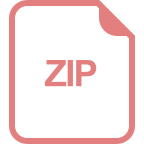
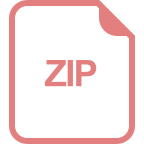
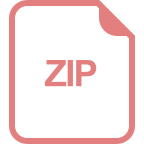
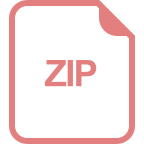
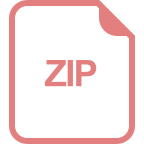
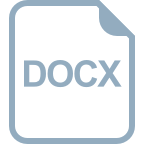