编写一个校验密码的弹窗
时间: 2024-03-26 20:41:05 浏览: 64
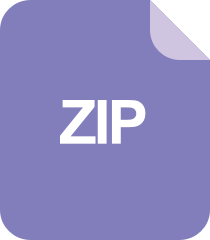
vue支付密码组件实现6位数字支付密码弹窗隐藏
好的,对于这个问题,我们可以通过 HTML、CSS 和 JavaScript 来编写一个校验密码的弹窗。具体步骤如下:
1. 在 HTML 文件中,创建一个按钮和弹窗的容器。可以使用 `<button>` 标签创建按钮,并给按钮设置一个唯一的 ID,例如 `open-modal`。使用 `<div>` 标签创建弹窗容器,并在其中创建一个表单,用于输入密码。表单中需要包含一个输入框和一个提交按钮。
```html
<button id="open-modal">点击弹窗</button>
<div id="modal" class="modal">
<div class="modal-content">
<h2>请输入密码</h2>
<form>
<input type="password" placeholder="请输入密码" id="password-input">
<button type="submit" id="submit-button">提交</button>
</form>
</div>
</div>
```
2. 在 CSS 文件中,设置弹窗容器和表单的样式。可以使用 `position: fixed` 和 `top: 0` 等属性将弹窗固定在页面上方,并使用 `display: none` 属性将其默认隐藏。还可以使用 `transform: translate(-50%, -50%)` 属性将弹窗居中显示。
```css
.modal {
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-color: rgba(0, 0, 0, 0.5);
display: none;
}
.modal-content {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
width: 300px;
background-color: white;
padding: 20px;
border-radius: 5px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.3);
}
input[type="password"] {
display: block;
width: 100%;
padding: 10px;
margin-bottom: 10px;
border: 1px solid #ccc;
border-radius: 5px;
}
button[type="submit"] {
display: block;
width: 100%;
padding: 10px;
background-color: #007bff;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
}
```
3. 在 JavaScript 文件中,为按钮添加点击事件,使其在点击时显示弹窗。同时,为表单添加提交事件,校验密码是否正确。如果密码正确,则关闭弹窗;否则,弹出提示信息。
```javascript
const openModalButton = document.getElementById("open-modal");
const modal = document.getElementById("modal");
const passwordInput = document.getElementById("password-input");
const submitButton = document.getElementById("submit-button");
openModalButton.addEventListener("click", function() {
modal.style.display = "block";
});
submitButton.addEventListener("click", function(event) {
event.preventDefault(); // 阻止表单默认提交行为
const password = passwordInput.value;
if (password === "123456") { // 校验密码是否正确
modal.style.display = "none"; // 关闭弹窗
} else {
alert("密码错误,请重新输入!"); // 弹出提示信息
}
});
```
完成上述步骤后,就可以实现一个校验密码的弹窗了。
阅读全文
相关推荐
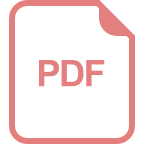
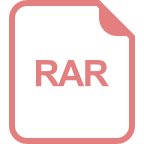
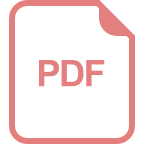
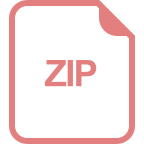
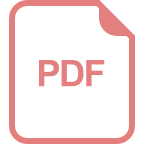
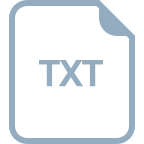
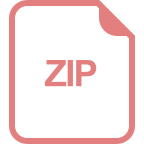
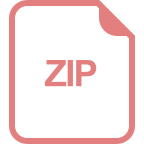
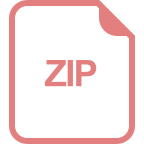
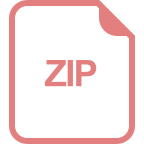
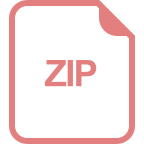
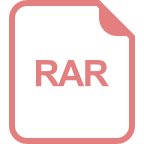
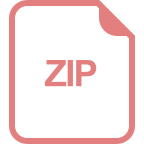
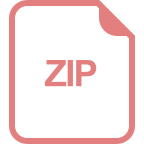