C#webclient下载整个目录
时间: 2024-12-24 12:36:33 浏览: 8
在C#中,使用`WebClient`类通常用于下载单个文件,而不是整个目录。如果需要下载整个目录,更合适的方式是通过FTP客户端库或者使用`Directory.CreateDirectory`配合循环遍历URL获取每个文件并下载。
但是,由于`WebClient`本身并不支持目录操作,你可以考虑使用第三方库如`FluentFTP`或者`SharpZipLib`等。下面是一个简单的示例,说明如何使用`FluentFTP`下载目录中的所有文件:
```csharp
using System;
using FluentFTP;
class Program
{
static async Task Main(string[] args)
{
string connectionString = "your_ftp_connection_string";
string downloadFolder = @"C:\path\to\download";
using var client = new FtpClient(connectionString);
await client.ConnectAsync();
if (!await client.ListDirectory())
throw new Exception("Failed to list directory contents");
// 获取目录下的文件列表
var files = (await client.ListDetails()).ToList();
foreach (var file in files)
{
try
{
// 假设所有的文件都在根目录下
string remotePath = file.FullName;
string localPath = Path.Combine(downloadFolder, remotePath.Substring(remotePath.LastIndexOf("/") + 1));
await client.DownloadAsync(remotePath, localPath);
Console.WriteLine($"Downloaded {localPath}");
}
catch (Exception ex)
{
Console.WriteLine($"Error downloading {remotePath}: {ex.Message}");
}
}
await client.DisconnectAsync();
}
}
```
注意:你需要替换`connectionString`为实际的FTP服务器连接字符串,并根据实际情况调整`ListDirectory`和`DownloadAsync`的路径处理。
阅读全文
相关推荐









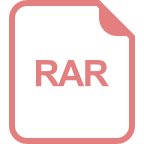








