python 倒计时
时间: 2023-09-23 09:07:18 浏览: 82
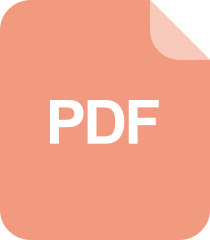
python实现倒计时小工具
Sure! Here's a Python code snippet for implementing a countdown:
```python
import time
def countdown(seconds):
while seconds > 0:
print(seconds)
time.sleep(1)
seconds -= 1
print("Time's up!")
countdown(10) # Replace 10 with the desired number of seconds
```
This code uses a `countdown` function that takes the number of seconds as an argument. It then loops through the countdown, printing each second and pausing for one second using the `time.sleep()` function. After the countdown reaches zero, it prints "Time's up!".
Feel free to adjust the duration by changing the argument passed to the `countdown` function.
阅读全文
相关推荐
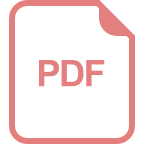

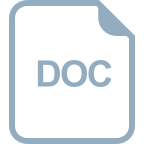













