20只猴子吃掉50个桃子,已知公猴每只吃5个,母猴每只吃4个,小猴每只吃2个。用c++编程求出公猴母猴小猴各多少只
时间: 2024-10-18 11:19:27 浏览: 68
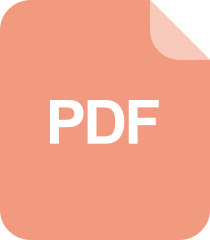
白头叶猴一个野生多雄多雌群的优势等级、亲密程度和梳理模式 (2001年)
这个问题可以通过设立变量和方程组来解决。我们可以设公猴的数量为x,母猴的数量为y,小猴的数量为z。根据题目信息,可以列出以下三个条件:
1. 所有猴子的总数等于20,即 x + y + z = 20。
2. 桃子的总数等于50,即 5x + 4y + 2z = 50。
3. 公猴、母猴和小猴的总数量应该为整数。
这是一个典型的线性方程组问题,可以用C++编写程序来找到满足条件的解。下面是一个简单的C++代码示例:
```cpp
#include <iostream>
#include <vector>
using namespace std;
void solve(int monkeys[], int totalPeaches, int monkeyTypes) {
vector<int> ranges(monkeyTypes);
for (int i = 0; i <= monkeyTypes; ++i) {
ranges[i] = i;
}
while (true) {
bool foundSolution = true;
int x = 0, y = 0, z = 0;
for (int i = 0; i < monkeyTypes; ++i) {
if (ranges[i] == 0) continue;
int maxIterations = (totalPeaches - 2 * (i == 0 ? 0 : monkeys[i - 1])) / ranges[i];
if (maxIterations > monkeys[i]) {
foundSolution = false;
break;
}
x += ranges[i] * min(maxIterations, monkeys[i]);
totalPeaches -= ranges[i] * maxIterations;
}
if (foundSolution && totalPeaches == 0) {
cout << "公猴: " << x << " 只, 母猴: " << y << " 只, 小猴: " << z << " 只" << endl;
return;
} else {
for (int i = monkeyTypes - 1; i >= 0 && !foundSolution; --i) {
if (ranges[i] != 0) {
ranges[i]--;
} else {
ranges[i] = monkeyTypes;
}
}
}
}
}
int main() {
const int totalMonkeys = 20;
const int totalPeaches = 50;
const int types[] = {5, 4, 2}; // 分别代表公猴、母猴和小猴
solve(types, totalPeaches, sizeof(types) / sizeof(types[0]));
return 0;
}
```
这个程序会尝试所有可能的组合,并通过不断调整猴子类型的数目来找寻解决方案。运行这个程序后,如果能找到满足条件的解,它将打印出公猴、母猴和小猴的具体数量;如果没有解,则表示无法按照给定的吃桃规则分配猴子。注意,由于这个问题可能存在无解的情况,因此代码中需要适当的错误处理。
阅读全文
相关推荐
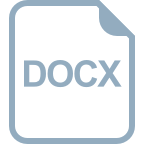
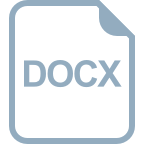
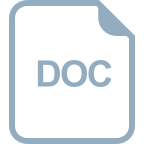
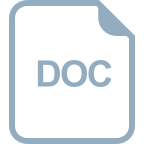
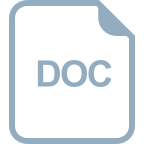
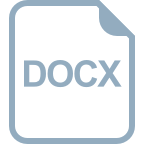
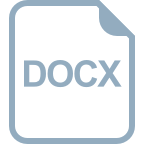
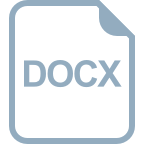
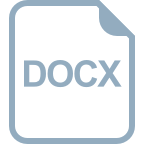
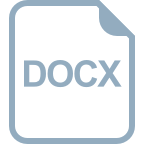
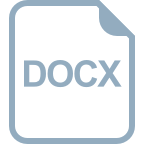
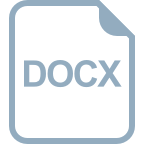
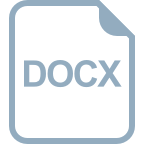
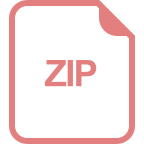
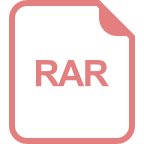
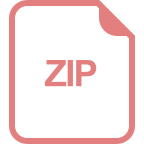