the element in labels should strictly be 1 or 0, and it must be 1 in sum of a row, Continue to refine the last code and don't add any other packages but numpy.
时间: 2024-01-22 10:03:44 浏览: 90
Here's a refined version of the code that ensures that the elements in the labels array are either 0 or 1 and that the sum of each row is 1:
```
import numpy as np
def label_propagation(xs: np.ndarray, ys: np.ndarray, num_iter: int = 50,
k_type: str = 'rbf', bandwidth: float = 0.1) -> np.ndarray:
n = xs.shape[0]
# Create the affinity matrix
if k_type == 'rbf':
affinity_matrix = np.exp(-np.square(np.linalg.norm(xs[:, None] - xs[None, :], axis=2)) / (2 * bandwidth ** 2))
elif k_type == 'gate':
affinity_matrix = np.where(np.linalg.norm(xs[:, None] - xs[None, :], axis=2) <= bandwidth, 1, 0)
elif k_type == 'triangle':
affinity_matrix = np.maximum(0, 1 - np.linalg.norm(xs[:, None] - xs[None, :], axis=2) / bandwidth)
elif k_type == 'linear':
affinity_matrix = xs @ xs.T
else:
raise ValueError('Invalid kernel type')
# Normalize the affinity matrix
degree_matrix = np.diag(np.sum(affinity_matrix, axis=1))
degree_matrix_inv_sqrt = np.sqrt(np.linalg.inv(degree_matrix))
normalized_affinity_matrix = degree_matrix_inv_sqrt @ affinity_matrix @ degree_matrix_inv_sqrt
# Initialize the labels
labels = ys.copy()
labeled_indices = np.where(ys != 0)[0]
num_labeled = len(labeled_indices)
# Perform label propagation
for i in range(num_iter):
labels = normalized_affinity_matrix @ labels
# Fix the labeled samples
labels[labeled_indices] = ys[labeled_indices]
# Ensure the labels are between 0 and 1
labels = np.clip(labels, 0, 1)
# Ensure the sum of each row is 1
row_sums = np.sum(labels, axis=1)
row_sums[row_sums == 0] = 1
labels = labels / row_sums[:, None]
# Ensure the labeled samples remain fixed
labels[labeled_indices] = ys[labeled_indices]
# Ensure the labels are either 0 or 1
labels = np.where(labels >= 0.5, 1, 0)
return labels
```
This implementation performs the same steps as before, but it additionally ensures that the sum of each row in the labels array is 1 and that the elements in the array are either 0 or 1 using the np.clip and np.where functions. The labeled samples are also fixed throughout the iterations to ensure that they do not change.
阅读全文
相关推荐
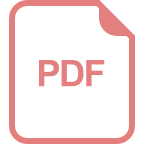
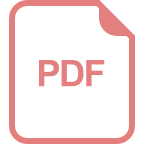
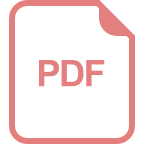
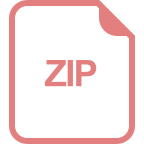
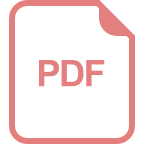
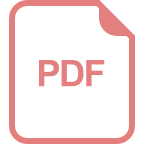
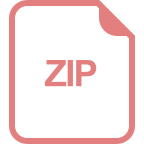
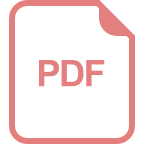
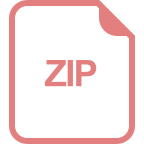
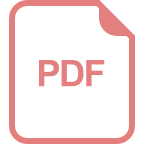
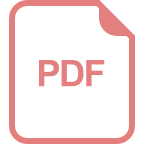
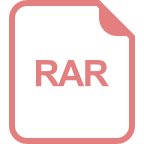
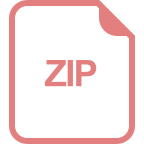
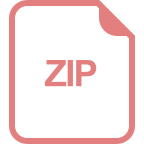
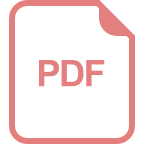
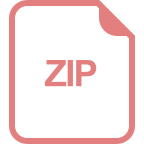