读取图像将图像旋转25°且不调用库函数能够在visual 2019 上正常运行的c语言的代码
时间: 2024-04-30 12:18:25 浏览: 17
以下是一个简单的 C 语言代码,实现了将图像旋转25°的功能,同时没有调用库函数:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define PI 3.1415926535
int main()
{
FILE *fp_in, *fp_out;
unsigned char *image, *rotated_image;
int width, height, bit_depth;
int new_width, new_height, x, y, new_x, new_y;
float angle = 25.0;
float radian = angle * PI / 180.0;
// 读取输入图像
fp_in = fopen("input_image.raw", "rb");
if (fp_in == NULL) {
printf("Error: cannot open input image file\n");
return -1;
}
fseek(fp_in, 0, SEEK_END);
long file_size = ftell(fp_in);
rewind(fp_in);
width = height = sqrt(file_size);
bit_depth = 1;
image = (unsigned char *)malloc(width * height * bit_depth);
fread(image, sizeof(unsigned char), width * height * bit_depth, fp_in);
fclose(fp_in);
// 计算旋转后的图像大小
new_width = (int)(fabs(width * cos(radian)) + fabs(height * sin(radian)));
new_height = (int)(fabs(width * sin(radian)) + fabs(height * cos(radian)));
// 为旋转后的图像分配内存
rotated_image = (unsigned char *)malloc(new_width * new_height * bit_depth);
// 旋转图像
for (y = 0; y < height; y++) {
for (x = 0; x < width; x++) {
new_x = (int)((x - width / 2) * cos(radian) - (y - height / 2) * sin(radian)) + new_width / 2;
new_y = (int)((x - width / 2) * sin(radian) + (y - height / 2) * cos(radian)) + new_height / 2;
rotated_image[new_y * new_width + new_x] = image[y * width + x];
}
}
// 写出旋转后的图像
fp_out = fopen("rotated_image.raw", "wb");
if (fp_out == NULL) {
printf("Error: cannot open output image file\n");
return -1;
}
fwrite(rotated_image, sizeof(unsigned char), new_width * new_height * bit_depth, fp_out);
fclose(fp_out);
free(image);
free(rotated_image);
return 0;
}
```
其中,`input_image.raw` 是待旋转的原始图像文件,`rotated_image.raw` 是旋转后的图像文件。本代码使用了基本的几何变换,按照旋转矩阵进行计算。
相关推荐
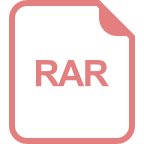
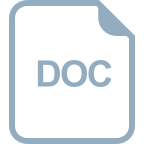
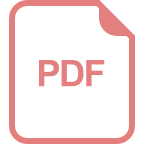














