//Clock.java import java.awt.*; import javax.swing.*; import java.util.*; //import Date and Locale import java.text.SimpleDateFormat; public class Clock extends JApplet implements Runnable { Thread clockThread; public void init() { //在JApplet的方法init 中,创建一线程命名为Clock clockThread = new Thread(this, "Clock-thread"); clockThread.start(); } //实现Runnable接口中的方法run,每隔一秒刷新时钟画面 public void run() { boolean flag=true; while (flag) { repaint(); try { clockThread.sleep(1000); } catch (InterruptedException e) { flag=false;} } //end of while } //end of run public void paint(Graphics g){ //JApplet的方法paint,显示当前时钟对象的值 super.paint(g); SimpleDateFormat formatter = new SimpleDateFormat (" hh:mm:ss ", Locale.getDefault()); Date currentDate = new Date(); String lastdate = formatter.format(currentDate); g.drawString(lastdate ,5, 10); } public void destroy() { //JApplet的方法 clockThread.interrupt(); } }
时间: 2024-02-14 18:09:51 浏览: 20
这是一个Java程序,用于显示当前时间的时钟程序,实现了JApplet和Runnable接口。
在程序中,首先导入了几个Java类库:
- java.awt.*
- javax.swing.*
- java.util.*
- java.text.SimpleDateFormat
然后创建了一个名为"Clock"的Java类,继承了JApplet,并实现了Runnable接口。
在init()方法中,创建了一个名为"Clock-thread"的线程,并启动该线程。
在run()方法中,使用repaint()方法每隔1秒钟刷新时钟画面,直到线程被中断。
在paint()方法中,使用SimpleDateFormat类获取当前时间,并将其格式化为"hh:mm:ss"的字符串,最后在画面上显示出来。
在destroy()方法中,使用interrupt()方法中断线程。
总体来说,这是一个简单的时钟程序,可以在Java Applet中运行。
相关问题
用中文注释一下代码://Clock.java import java.awt.*; import javax.swing.*; import java.util.*; //import Date and Locale import java.text.SimpleDateFormat; public class Clock extends JApplet implements Runnable { Thread clockThread; public void init() { //在JApplet的方法init 中,创建一线程命名为Clock clockThread = new Thread(this, "Clock-thread"); clockThread.start(); } //实现Runnable接口中的方法run,每隔一秒刷新时钟画面 public void run() { boolean flag=true; while (flag) { repaint(); try { clockThread.sleep(1000); } catch (InterruptedException e) { flag=false;} } //end of while } //end of run public void paint(Graphics g){ //JApplet的方法paint,显示当前时钟对象的值 super.paint(g); SimpleDateFormat formatter = new SimpleDateFormat (" hh:mm:ss ", Locale.getDefault()); Date currentDate = new Date(); String lastdate = formatter.format(currentDate); g.drawString(lastdate ,5, 10); } public void destroy() { //JApplet的方法 clockThread.interrupt(); } }
//Clock.java
//导入需要使用的类库
import java.awt.*;
import javax.swing.*;
import java.util.*;
import java.text.SimpleDateFormat;
//定义一个类Clock,继承自JApplet类,并实现Runnable接口
public class Clock extends JApplet implements Runnable {
//声明一个线程对象clockThread
Thread clockThread;
//在JApplet的方法init中,初始化clockThread线程并启动
public void init() {
clockThread = new Thread(this, "Clock-thread");
clockThread.start();
}
//实现Runnable接口中的方法run,每隔一秒刷新时钟画面
public void run() {
boolean flag=true;
while (flag) {
repaint(); //调用repaint()方法,刷新时钟画面
try {
clockThread.sleep(1000); //线程休眠一秒钟
} catch (InterruptedException e) {
flag=false;
}
} //end of while
} //end of run
//实现JApplet的方法paint,显示当前时钟对象的值
public void paint(Graphics g){
super.paint(g); //调用父类的paint()方法
SimpleDateFormat formatter = new SimpleDateFormat (" hh:mm:ss ", Locale.getDefault()); //创建日期格式化对象
Date currentDate = new Date(); //获取当前时间
String lastdate = formatter.format(currentDate); //将当前时间格式化
g.drawString(lastdate ,5, 10); //在指定位置绘制时间字符串
}
//实现JApplet的方法destroy,中断线程
public void destroy() {
clockThread.interrupt(); //中断线程
}
}
package T13; //Clock.java import java.awt.*; import javax.swing.*; import java.util.*; //import Date and Locale import java.text.SimpleDateFormat; public class Clock extends JApplet implements Runnable { Thread clockThread; public void init() { //在JApplet的方法init 中,创建一线程命名为Clock clockThread = new Thread(this, "Clock-thread"); clockThread.start(); } //实现Runnable接口中的方法run,每隔一秒刷新时钟画面 public void run() { boolean flag=true; while (flag) { repaint(); try { clockThread.sleep(1000); } catch (InterruptedException e) { flag=false;} } //end of while } //end of run public void paint(Graphics g){ //JApplet的方法paint,显示当前时钟对象的值 super.paint(g); SimpleDateFormat formatter = new SimpleDateFormat (" hh:mm:ss ", Locale.getDefault()); Date currentDate = new Date(); String lastdate = formatter.format(currentDate); g.drawString(lastdate ,5, 10); } public void destroy() { //JApplet的方法 clockThread.interrupt(); } }
好的,下面是添加注释后的代码:
```java
package T13; // 定义包名
import java.awt.*; // 导入 AWT 组件
import javax.swing.*; // 导入 Swing 组件
import java.util.*; // 导入 Java 的工具类
// 导入日期格式化工具类
import java.text.SimpleDateFormat;
public class Clock extends JApplet implements Runnable { // 定义 Clock 类,并实现 Runnable 接口
Thread clockThread; // 定义线程变量
public void init() { // 重写 JApplet 的 init() 方法
clockThread = new Thread(this, "Clock-thread"); // 创建线程对象
clockThread.start(); // 启动线程
}
public void run() { // 实现 Runnable 接口的 run() 方法
boolean flag = true; // 定义标志变量
while (flag) { // 如果标志变量为 true,就一直循环
repaint(); // 调用 repaint() 方法,刷新时钟的画面
try {
clockThread.sleep(1000); // 让线程休眠 1 秒
} catch (InterruptedException e) {
flag = false; // 如果线程被中断,将标志变量设为 false,跳出循环
}
}
}
public void paint(Graphics g) { // 重写 JApplet 的 paint() 方法
super.paint(g); // 调用父类的 paint() 方法
SimpleDateFormat formatter = new SimpleDateFormat(" hh:mm:ss ", Locale.getDefault()); // 创建日期格式化对象
Date currentDate = new Date(); // 创建日期对象
String lastdate = formatter.format(currentDate); // 调用格式化对象的 format() 方法,将日期格式化成字符串
g.drawString(lastdate, 5, 10); // 在画布上显示字符串
}
public void destroy() { // 重写 JApplet 的 destroy() 方法
clockThread.interrupt(); // 中断线程
}
}
```
相关推荐
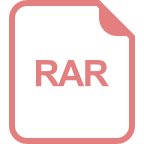
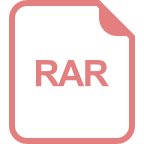











