用C语言构造两个带有表头节点的有序单链表La 、Lb. 编写程序实现将La,Lb 合并成一个有序单链表Lc
时间: 2024-05-12 22:16:48 浏览: 103
好的,我知道了。下面是程序的实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表结构体
typedef struct node {
int data; // 数据域
struct node *next; // 指针域
} Node, *LinkedList;
// 创建带有表头节点的有序单链表
LinkedList createList(int n) {
Node *head = (Node *) malloc(sizeof(Node)); // 创建头节点
head->data = -1; // 头节点不存储数据
head->next = NULL;
LinkedList p = head; // 指向头节点
// 依次输入 n 个元素,创建单链表
for (int i = 0; i < n; i++) {
Node *node = (Node *) malloc(sizeof(Node));
scanf("%d", &node->data);
// 找到要插入的位置
while (p->next != NULL && p->next->data < node->data) {
p = p->next;
}
// 插入新结点
node->next = p->next;
p->next = node;
p = head; // 恢复指向头节点
}
return head;
}
// 合并两个有序单链表为一个有序单链表
LinkedList merge(LinkedList La, LinkedList Lb) {
Node *pa = La->next, *pb = Lb->next; // pa 和 pb 分别指向 La 和 Lb 的第一个元素
Node *tail = La; // tail 指向 La 的尾节点
LinkedList Lc = La; // Lc 指向 La
while (pa != NULL && pb != NULL) {
if (pa->data < pb->data) {
tail->next = pa;
tail = pa;
pa = pa->next;
} else {
tail->next = pb;
tail = pb;
pb = pb->next;
}
}
if (pa != NULL) {
tail->next = pa;
} else {
tail->next = pb;
}
return Lc;
}
// 输出单链表
void printList(LinkedList L) {
printf("List: ");
Node *p = L->next;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
int main() {
printf("请输入 La 的元素个数:");
int n;
scanf("%d", &n);
LinkedList La = createList(n);
printList(La);
printf("\n请输入 Lb 的元素个数:");
scanf("%d", &n);
LinkedList Lb = createList(n);
printList(Lb);
LinkedList Lc = merge(La, Lb);
printf("\n合并后的有序单链表 Lc:");
printList(Lc);
return 0;
}
```
希望能够帮到你!
阅读全文
相关推荐












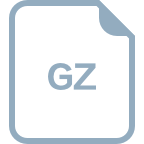



