C++ 将string类型转为short或int型
时间: 2023-04-09 13:01:58 浏览: 259
可以使用stoi函数将string类型转为int型,也可以使用stol函数将string类型转为long型,使用stoll函数将string类型转为long long型,使用stoul函数将string类型转为unsigned long型,使用stoull函数将string类型转为unsigned long long型,使用stoi函数将string类型转为short型,使用stoul函数将string类型转为unsigned short型。
相关问题
string转short
要将字符串转换为short类型,可以使用以下方法:
```c
public short[] stringToShort(String inString, out short[] outShort) {
if (inString.Length % 2 == 1) {
inString = inString + " ";
}
char[] bufChar = inString.ToCharArray();
byte[] oufByte = Encoding.Default.GetBytes(bufChar);
byte[] bufByte = new byte = oufByte[i];
bufByte = oufByte[i + 1];
outShort[j = BitConverter.ToInt16(bufByte, 0);
}
return outShort;
}
private void button1_Click(object sender, EventArgs e) {
short[] arr2 = new short<span class="em">1</span><span class="em">2</span>
#### 引用[.reference_title]
- *1* [C# STRING TO SHORT[]方法](https://blog.csdn.net/weixin_43120117/article/details/103005937)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
- *2* [C++定义函数模板代码,可以编写通用的函数来处理多种类型的容器](https://download.csdn.net/download/weixin_44609920/88222478)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
c++实现把一个short类型的值写入buffer中和从buffer中读出一个short类型值,请用二进制和文本实现
实现把一个short类型的值写入buffer中和从buffer中读出一个short类型值的示例代码如下:
二进制实现:
```c++
#include <iostream>
#include <cstring>
using namespace std;
int main() {
short value = 1234;
char buffer[sizeof(short)]; // buffer的大小为short类型的大小
// 将short类型的值写入buffer中
memcpy(buffer, &value, sizeof(value));
// 从buffer中读出short类型的值
short result;
memcpy(&result, buffer, sizeof(result));
cout << "value: " << value << endl; // 输出原始值
cout << "result: " << result << endl; // 输出从buffer中读出的值
return 0;
}
```
文本实现:
```c++
#include <iostream>
#include <sstream>
using namespace std;
int main() {
short value = 1234;
ostringstream oss; // 使用ostringstream将short类型的值转换成字符串
// 将short类型的值转换成字符串并写入到ostringstream中
oss << value;
string str = oss.str(); // 获取ostringstream中的字符串
short result = stoi(str); // 使用stoi将字符串转换成short类型的值
cout << "value: " << value << endl; // 输出原始值
cout << "result: " << result << endl; // 输出从字符串中读出的值
return 0;
}
```
相关推荐
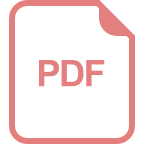
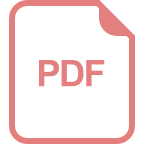
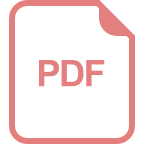












