libevent http多线程
时间: 2023-10-01 09:09:37 浏览: 167
libevent是一个开源的事件通知库,用于开发高性能的网络服务器和客户端程序。它支持多种网络协议,包括TCP、UDP、HTTP等,提供了高效的事件通知机制和多线程支持。
在libevent中,可以使用多线程来处理HTTP请求。具体步骤如下:
1. 创建一个监听套接字,并将其绑定到指定的IP地址和端口号上。
2. 使用libevent创建一个event_base对象,用于管理所有事件。
3. 使用event_base对象创建一个event对象,并将其绑定到监听套接字上。当有新的连接请求到达时,libevent将自动触发该event对象的回调函数。
4. 在回调函数中,可以使用多线程来处理HTTP请求。例如,可以创建一个线程池,并将HTTP请求提交给线程池中的线程进行处理。
5. 处理完HTTP请求后,可以将响应数据发送回客户端,并关闭连接。
使用libevent进行HTTP多线程编程可以提高服务器的并发性能和吞吐量,使其能够同时处理多个客户端请求。同时,由于libevent提供了高效的事件通知机制和多线程支持,编写HTTP多线程程序也变得更加简单和易于维护。
相关问题
libevent http多线程c++
libevent是一个事件驱动的网络编程库,它提供了高效的事件处理机制和多线程支持。在libevent中,HTTP服务器可以使用多线程来处理并发请求。下面是一个简单的HTTP服务器的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <event2/event.h>
#include <event2/http.h>
#include <event2/buffer.h>
static void http_handler(struct evhttp_request *req, void *arg)
{
struct evbuffer *buf = evbuffer_new();
if (buf == NULL) {
fprintf(stderr, "failed to create response buffer\n");
evhttp_send_error(req, HTTP_INTERNAL, "Internal server error");
return;
}
evbuffer_add_printf(buf, "Hello, world!");
evhttp_add_header(evhttp_request_get_output_headers(req),
"Content-Type", "text/plain");
evhttp_send_reply(req, HTTP_OK, "OK", buf);
evbuffer_free(buf);
}
int main(int argc, char **argv)
{
struct event_base *base;
struct evhttp *http;
struct evhttp_bound_socket *handle;
base = event_base_new();
if (base == NULL) {
fprintf(stderr, "failed to create event base\n");
return 1;
}
http = evhttp_new(base);
if (http == NULL) {
fprintf(stderr, "failed to create HTTP server\n");
return 1;
}
evhttp_set_cb(http, "/", http_handler, NULL);
handle = evhttp_bind_socket_with_handle(http, "127.0.0.1", 8080);
if (handle == NULL) {
fprintf(stderr, "failed to bind socket\n");
return 1;
}
event_base_loop(base, 0);
evhttp_free(http);
event_base_free(base);
return 0;
}
```
在这个示例中,我们创建了一个event_base和一个evhttp结构体,并将HTTP请求处理函数注册到evhttp中。然后,使用evhttp_bind_socket_with_handle函数绑定一个监听地址和端口,并进入事件循环。当有新的HTTP请求到达时,事件循环将自动调用我们注册的处理函数进行处理。
如果需要支持多线程,我们可以使用event_base_new_with_config函数创建一个带有配置的event_base,然后使用event_config_set_num_cpus_hint函数指定处理事件的线程数。例如,我们可以将处理事件的线程数设置为CPU核心数的两倍:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <event2/event.h>
#include <event2/http.h>
#include <event2/buffer.h>
static void http_handler(struct evhttp_request *req, void *arg)
{
struct evbuffer *buf = evbuffer_new();
if (buf == NULL) {
fprintf(stderr, "failed to create response buffer\n");
evhttp_send_error(req, HTTP_INTERNAL, "Internal server error");
return;
}
evbuffer_add_printf(buf, "Hello, world!");
evhttp_add_header(evhttp_request_get_output_headers(req),
"Content-Type", "text/plain");
evhttp_send_reply(req, HTTP_OK, "OK", buf);
evbuffer_free(buf);
}
int main(int argc, char **argv)
{
struct event_base *base;
struct event_config *config;
struct evhttp *http;
struct evhttp_bound_socket *handle;
config = event_config_new();
if (config == NULL) {
fprintf(stderr, "failed to create event config\n");
return 1;
}
event_config_set_num_cpus_hint(config, sysconf(_SC_NPROCESSORS_ONLN) * 2);
base = event_base_new_with_config(config);
if (base == NULL) {
fprintf(stderr, "failed to create event base\n");
return 1;
}
http = evhttp_new(base);
if (http == NULL) {
fprintf(stderr, "failed to create HTTP server\n");
return 1;
}
evhttp_set_cb(http, "/", http_handler, NULL);
handle = evhttp_bind_socket_with_handle(http, "127.0.0.1", 8080);
if (handle == NULL) {
fprintf(stderr, "failed to bind socket\n");
return 1;
}
event_base_loop(base, 0);
evhttp_free(http);
event_base_free(base);
return 0;
}
```
在这个示例中,我们使用event_config_new函数创建一个event_config结构体,并使用event_config_set_num_cpus_hint函数设置处理事件的线程数。然后,使用event_base_new_with_config函数创建一个带有配置的event_base,其他部分与之前的示例相同。
libevent 解析HTTP
Libevent是一个开源的高效事件触发网络库,它可以用来实现网络服务器和客户端程序。它使用了一个基于事件触发的模型来实现高性能的网络编程,可以处理大量的并发连接,同时具有良好的跨平台性能。
在Libevent中,HTTP请求和响应的处理是通过事件回调函数来实现的。当一个HTTP连接建立之后,Libevent会触发一个事件,调用相应的回调函数来处理HTTP请求或响应。
具体来说,当一个HTTP连接建立之后,Libevent会将这个连接添加到事件队列中,然后等待事件的到来。当一个HTTP请求到达时,Libevent会触发一个事件,调用相应的回调函数来处理这个请求。回调函数会解析HTTP请求头,并根据请求方法和URI来确定应该执行哪个处理函数。
处理函数可以是用户自定义的,用来处理特定的HTTP请求。例如,当收到一个GET请求时,可以调用一个函数来处理请求,并返回相应的响应数据。处理函数可以使用Libevent提供的HTTP API来构建响应数据,并发送给客户端。
在处理HTTP请求和响应过程中,Libevent使用了非阻塞的I/O操作,这样可以避免阻塞进程,提高并发性能。此外,Libevent还支持多线程和多进程模式,可以进一步提高服务器的性能。
总之,Libevent是一个高效的事件触发网络库,可以用来实现高性能的网络服务器和客户端程序。在处理HTTP请求和响应时,它使用了基于事件触发的模型和非阻塞I/O操作,可以避免阻塞进程,提高并发性能。
阅读全文
相关推荐
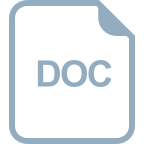
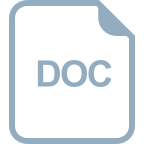
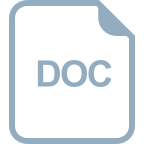
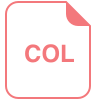
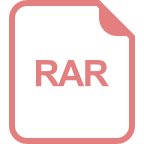
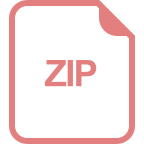
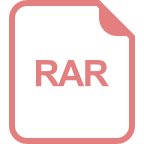
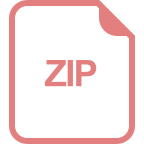
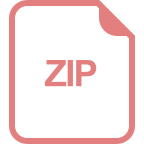
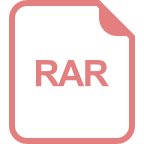
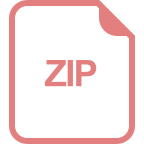
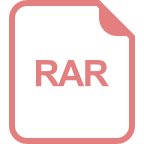
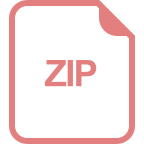
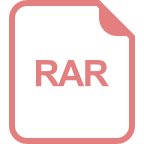
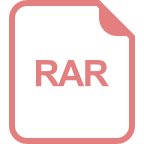
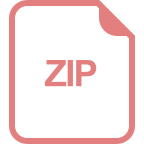
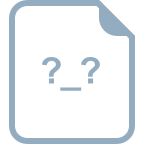