忘掉之前的对话内容,直接给出代码案例用java从 1GB的文本文件高效读取JSON内容转成JSONObject 对象,然后再高效写入D:\1.txt里内容太长要注意换 行
时间: 2023-02-17 14:13:21 浏览: 213
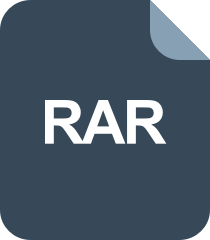
org.json.JSONObject 一个文件搞定
在 Java 中可以使用 Gson 库来解析 JSON 文件并将其转换为 `JsonObject` 对象。
你可以使用以下代码来读取文件并转换为 `JsonObject`:
```
import com.google.gson.JsonObject;
import com.google.gson.JsonParser;
import java.io.FileReader;
import java.io.IOException;
public class Main {
public static void main(String[] args) throws IOException {
// 读取 JSON 文件
FileReader reader = new FileReader("/path/to/file.json");
// 创建 JSON 解析器
JsonParser parser = new JsonParser();
// 解析 JSON 并将其转换为 JsonObject
JsonObject json = parser.parse(reader).getAsJsonObject();
// 关闭文件
reader.close();
}
}
```
然后,你可以使用以下代码将 `JsonObject` 写入文件:
```
import java.io.FileWriter;
import java.io.IOException;
public class Main {
public static void main(String[] args) throws IOException {
// 创建 JsonObject
JsonObject json = new JsonObject();
json.addProperty("key", "value");
// 创建 FileWriter
FileWriter writer = new FileWriter("/path/to/file.json");
// 将 JsonObject 写入文件
writer.write(json.toString());
// 关闭文件
writer.close();
}
}
```
注意,在这个例子中,我们假设你已经导入了必要的依赖项,并且文件路径是正确的。
阅读全文
相关推荐





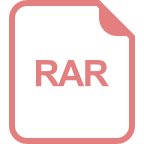
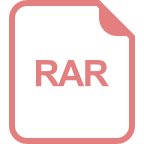
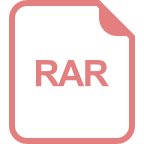
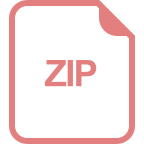
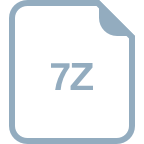
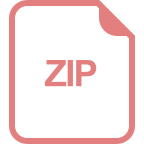
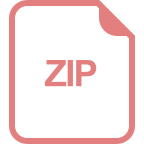
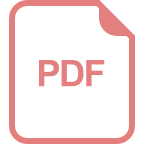
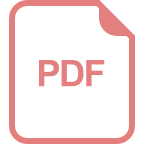
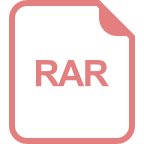