C语言写一个打印很大非负整数阶乘的函数
时间: 2024-10-18 22:11:13 浏览: 22
在C语言中,计算大整数阶乘通常会涉及到溢出问题,因为整型数据类型的大小有限。为了处理这种情况,可以使用递归算法并借助于字符串来存储中间结果,然后转换回整数。下面是一个简单的例子:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 助记符类型用于存储临时的数字字符串
typedef struct {
char num[100];
int size; // 存储数字的字符数组长度
} BigInt;
// 函数用来相乘两个BigInt
void multiply(BigInt* a, BigInt* b) {
int carry = 0;
for (int i = a->size - 1, j = b->size - 1; i >= 0 || j >= 0; --i, --j) {
int product = (a->num[i] - '0') * (b->num[j] - '0') + carry;
carry = product / 10;
a->num[i] = product % 10 + '0';
if (carry > 0 && (i == 0 || a->num[i] != '0')) {
a->num = realloc(a->num, sizeof(char) * (a->size + 2));
++a->size;
}
}
if (carry > 0) {
a->num = realloc(a->num, sizeof(char) * (a->size + 1));
++a->size;
a->num[a->size - 1] = carry + '0';
}
}
// 计算阶乘并返回结果的BigInt
BigInt factorial(BigInt n) {
BigInt result = {0, 1}; // 初始化结果为1
if (n.size > 1) {
multiply(&result, &BigInt{'1'}); // 处理基本情况 n=1时直接为1
for (int i = 2; i <= n.num[0] - '0'; ++i) {
multiply(&result, &BigInt{itoa(i, NULL, 10)});
}
}
return result;
}
// 将BigInt转换为整数并打印
void printBigInt(BigInt n) {
printf("%d", n.num[0] - '0');
if (n.size > 1) {
printf(".");
for (int i = 1; i < n.size; ++i) {
printf("%d", n.num[i] - '0');
}
}
}
int main() {
BigInt fact;
scanf("%s", fact.num);
fact.size = strlen(fact.num);
fact = factorial(fact);
printBigInt(fact);
printf("\n");
free(fact.num);
return 0;
}
```
请注意,这个示例仅适用于输入的小范围非负整数,如果需要处理更大的数值,可以考虑使用专门的大数库如GMP。
阅读全文
相关推荐






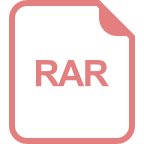
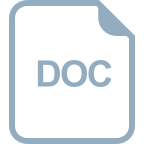









