html5 拖放跳棋游戏
时间: 2023-12-25 13:28:04 浏览: 166
以下是一个基于HTML5拖放API和JavaScript/Vue.js的跳棋游戏的示例:
```html
<!DOCTYPE html>
<html>
<head>
<title>HTML5拖放跳棋游戏</title>
<style>
.board {
display: flex;
flex-wrap: wrap;
width: 400px;
height: 400px;
border: 1px solid black;
}
.square {
width: 50px;
height: 50px;
box-sizing: border-box;
border: 1px solid black; display: flex;
align-items: center;
justify-content: center; font-size: 30px;
font-weight: bold;
cursor: pointer;
}
.square.white {
background-color: white;
}
.square.black {
background-color: gray;
}
.piece {
width: 40px;
height: 40px;
border-radius: 50%;
background-color: red;
cursor: pointer;
}
.piece.selected {
background-color: yellow;
}
.piece.king {
background-color: blue;
}
.chat {
width: 400px;
height: 200px;
border: 1px solid black;
overflow-y: scroll;
padding: 10px;
}
.input {
width: 100%;
margin-top: 10px;
display: flex;
}
.input input {
flex-grow: 1;
margin-right: 10px;
}
.input button {
width: 80px;
cursor: pointer;
}
</style>
</head>
<body>
<div id="app">
<div class="board">
<div v-for="(square, index) in squares" :key="index" :class="['square', square.color, {selected: selectedSquare === index}]" @click="selectSquare(index)">
<div v-if="square.piece" :class="['piece', square.piece.color, {king: square.piece.king}]" @dragstart="dragStart(index, $event)" draggable="true"></div>
</div>
</div>
<div class="chat">
<div v-for="(message, index) in messages" :key="index">{{message}}</div>
</div>
<div class="input">
<input type="text" v-model="newMessage" @keyup.enter="sendMessage">
<button @click="sendMessage">发送</button>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
<script>
const boardSize = 8;
const initialBoard = [
' ', 'b', ' ', 'b', ' ', 'b', ' ', 'b',
'b', ' ', 'b', ' ', 'b', ' ', 'b', ' ',
' ', 'b', ' ', 'b', ' ', 'b', ' ', 'b',
' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',
' ', ' ', ' ', ' ', ' ', ' ', ' ', ' ',
'w', ' ', 'w', ' ', 'w', ' ', 'w', ' ',
' ', 'w', ' ', 'w', ' ', 'w', ' ', 'w',
'w', ' ', 'w', ' ', 'w', ' ', 'w', ' '
];
const app = new Vue({
el: '#app',
data: {
squares: [],
selectedSquare: null,
messages: [],
newMessage: ''
},
created() {
this.resetBoard();
},
methods: {
resetBoard() {
this.squares = [];
for (let i = 0; i < boardSize * boardSize; i++) {
const row = Math.floor(i / boardSize);
const col = i % boardSize;
const color = (row + col) % 2 === 0 ? 'white' : 'black';
const piece = initialBoard[i] !== ' ' ? {color: initialBoard[i], king: false} : null;
this.squares.push({color, piece});
}
},
selectSquare(index) {
if (this.selectedSquare === null) {
if (this.squares[index].piece) {
this.selectedSquare = index;
}
} else {
if (this.isValidMove(this.selectedSquare, index)) {
this.movePiece(this.selectedSquare, index);
this.selectedSquare = null;
} else {
this.selectedSquare = null;
}
}
},
isValidMove(from, to) {
const piece = this.squares[from].piece;
if (!piece) {
return false;
}
const dx = Math.abs(to % boardSize - from % boardSize);
const dy = Math.abs(Math.floor(to / boardSize) - Math.floor(from / boardSize));
if (dx !== dy) {
return false;
}
if (dx === 1 && !piece.king) {
if (piece.color === 'w' && to < from) {
return false;
}
if (piece.color === 'b' && to > from) {
return false;
}
}
const jumpIndex = this.getJumpIndex(from, to);
if (jumpIndex !== null) {
if (this.squares[jumpIndex].piece || (piece.color === 'w' && jumpIndex < to) || (piece.color === 'b' && jumpIndex > to)) {
return false;
}
return true;
}
return false;
},
getJumpIndex(from, to) {
const piece = this.squares[from].piece;
if (!piece) {
return null;
}
const dx = to % boardSize - from % boardSize;
const dy = Math.floor(to / boardSize) - Math.floor(from / boardSize);
if (Math.abs(dx) !== Math.abs(dy) || Math.abs(dx) !== 2) {
return null;
}
const jumpIndex = from + dx / 2 + dy / 2 * boardSize;
if (this.squares[jumpIndex].color !== 'black' || this.squares[jumpIndex].piece) {
return null;
}
return jumpIndex;
},
movePiece(from, to) {
const piece = this.squares[from].piece;
this.squares[from].piece = null;
this.squares[to].piece = piece;
if (piece.color === 'w' && to >= boardSize * (boardSize - 1)) {
piece.king = true;
}
if (piece.color === 'b' && to < boardSize) {
piece.king = true;
}
},
dragStart(index, event) {
if (this.squares[index].piece) {
event.dataTransfer.setData('text/plain', index);
}
},
dragOver(index, event) {
event.preventDefault();
},
drop(index, event) {
event.preventDefault();
const from = event.dataTransfer.getData('text/plain');
if (this.isValidMove(from, index)) {
this.movePiece(from, index);
this.selectedSquare = null;
}
},
sendMessage() {
if (this.newMessage.trim() !== '') {
this.messages.push(this.newMessage.trim());
this.newMessage = '';
}
}
}
});
</script>
</body>
</html>
```
该示例使用Vue.js框架构建了一个8x8的跳棋游戏,玩家可以通过拖放棋子来移动它们。游戏还包括一个实时聊天功能,玩家可以在游戏中聊天。
阅读全文
相关推荐
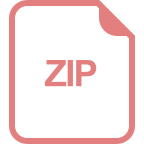
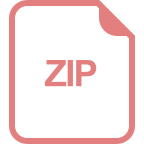
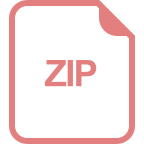
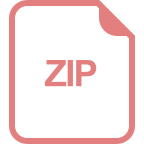
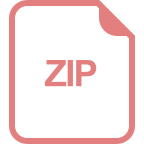
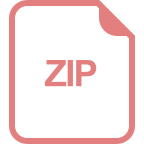