决策树c语言实现代码
时间: 2024-10-17 21:02:27 浏览: 97
决策树是一种常见的机器学习算法,用于分类和回归问题。在C语言中实现完整的决策树可能会比较复杂,因为C语言主要用于系统级编程,而不是数据处理和机器学习。然而,你可以使用一些库,比如libsvm或mlpack,它们提供了C语言接口的机器学习工具,其中包括决策树。
如果你想要简单的理解决策树的工作原理并手动构建一个基本版本,你可以编写递归函数来模拟节点划分、特征选择等步骤。下面是一个非常基础的示例,仅适用于二元分类问题:
```c
#include <stdio.h>
typedef struct Node {
int feature_index; // 划分的特征索引
double threshold; // 划分的阈值
char class_label; // 当前节点预测的类别
struct Node* left; // 左分支
struct Node* right; // 右分支
} Node;
// 构建一个决策树节点的基本函数
Node* create_node(int feature, double threshold, char label) {
Node* node = (Node*)malloc(sizeof(Node));
node->feature_index = feature;
node->threshold = threshold;
node->class_label = label;
node->left = NULL;
node->right = NULL;
return node;
}
// 简单决策树的示例,仅用于演示,实际应用会更复杂
void simple_decision_tree(Node* root, int data[], int n, char labels[]) {
// 这里只是一个简化版的划分逻辑,实际需要根据特征值和数据分布进行
if (n <= 1 || !labels[n - 1]) {
root->class_label = data[n - 1]; // 样本集中最后一个作为结果
} else {
// 选择最大差异度的特征和阈值
int max_diff = -1;
double best_threshold = -1.0;
for (int i = 0; i < n; ++i) {
double val = data[i];
if (val > best_threshold && labels[i] != labels[n - 1]) {
max_diff = i;
best_threshold = val;
}
}
if (max_diff == -1) { // 如果所有样本同类别,可以结束递归
root->class_label = labels[n - 1];
} else {
Node* left = create_node(max_diff, best_threshold, labels[max_diff]);
Node* right = create_node(-1, -1, labels[n - 1]); // 右分支标记无法继续划分
root->left = left;
root->right = right;
simple_decision_tree(left, &data[0], max_diff, labels);
simple_decision_tree(right, &data[max_diff + 1], n - max_diff - 1, labels + max_diff + 1);
}
}
}
// 使用示例
int main() {
// 假设你已经有了一个预处理好的数据集和标签数组
int data[] = {..};
char labels[] = {'A', 'B', ...};
Node* root = create_node(0, 50, '\0'); // 初始化根节点
simple_decision_tree(root, data, sizeof(data) / sizeof(data[0]), labels);
// 以此类推,你可以添加评估、预测等功能
return 0;
}
```
请注意,这个示例仅是个简化的起点,真实世界的应用通常会涉及更多的细节,如特征选择、剪枝、交叉验证等。而且,在实际项目中,建议使用专门的机器学习库,如Scikit-Learn(Python)、XGBoost(C++)或LightGBM(C++),它们有优化过的C语言实现,并提供了丰富的API和功能。
阅读全文
相关推荐
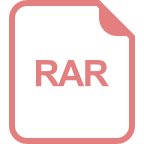
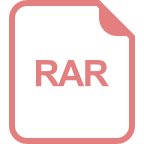
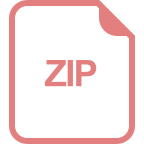


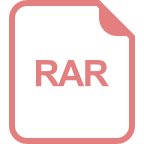
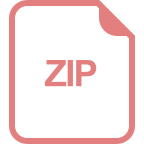
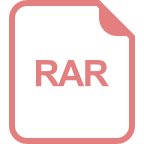
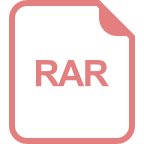
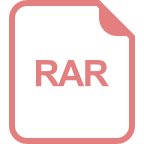
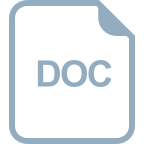
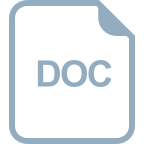
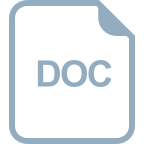
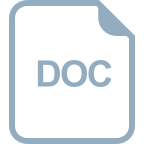
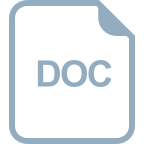
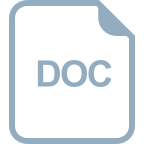