用Java编程实现对不同的数进行排序输出。在IntSort 类中定义3 个同名的方法sort。利用重载此方法可以处理两个数的排序,三个数的排序以及数组的排序。,当程序要实现多个相近的功能时,就给相应的方法起一个共同的名字,用不同的参数代表不同的功能。写出代码
时间: 2024-03-05 21:53:52 浏览: 59
好的,请看下面的Java代码实现:
```
public class IntSort {
// 对两个数排序
public void sort(int a, int b) {
if (a > b) {
int temp = a;
a = b;
b = temp;
}
System.out.println(a + " " + b);
}
// 对三个数排序
public void sort(int a, int b, int c) {
if (a > b) {
int temp = a;
a = b;
b = temp;
}
if (a > c) {
int temp = a;
a = c;
c = temp;
}
if (b > c) {
int temp = b;
b = c;
c = temp;
}
System.out.println(a + " " + b + " " + c);
}
// 对数组排序
public void sort(int[] arr) {
for (int i = 0; i < arr.length - 1; i++) {
for (int j = 0; j < arr.length - 1 - i; j++) {
if (arr[j] > arr[j + 1]) {
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
for (int num : arr) {
System.out.print(num + " ");
}
}
// 测试
public static void main(String[] args) {
IntSort intSort = new IntSort();
intSort.sort(2, 1);
intSort.sort(5, 3, 8);
int[] arr = {8, 3, 5, 1, 2};
intSort.sort(arr);
}
}
```
在 IntSort 类中定义了三个同名的方法 sort,利用重载来实现对两个数的排序、三个数的排序以及数组的排序。在 sort(int a, int b) 方法中,如果 a 大于 b,就交换两个数的位置。在 sort(int a, int b, int c) 方法中,先将三个数中最小的数放到第一个位置,然后将剩下的两个数进行排序。在 sort(int[] arr) 方法中,使用冒泡排序对数组进行排序,最后输出排序后的数组。
阅读全文
相关推荐

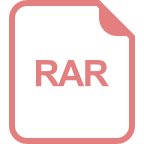













