nodejs中使用mysql做分页
时间: 2024-03-03 12:47:11 浏览: 67
在Node.js中使用MySQL做分页可以按照以下步骤进行:
1. 安装mysql包
使用npm命令安装mysql包:
```
npm install mysql
```
2. 连接MySQL数据库
使用以下代码连接MySQL数据库:
```javascript
const mysql = require('mysql');
const connection = mysql.createConnection({
host: 'localhost',
user: 'root',
password: 'password',
database: 'database_name'
});
connection.connect();
```
3. 查询总记录数
使用以下代码查询总记录数:
```javascript
const sql = 'SELECT COUNT(*) as total FROM table_name';
connection.query(sql, function(error, results, fields) {
if (error) throw error;
const total = results[0].total;
console.log(total);
});
```
4. 查询分页数据
使用以下代码查询分页数据:
```javascript
const pageSize = 10;
const currentPage = 1;
const offset = (currentPage - 1) * pageSize;
const sql = `SELECT * FROM table_name LIMIT ${offset}, ${pageSize}`;
connection.query(sql, function(error, results, fields) {
if (error) throw error;
console.log(results);
});
```
其中,pageSize为每页记录数,currentPage为当前页码,offset为偏移量。
5. 关闭连接
最后使用以下代码关闭连接:
```javascript
connection.end();
```
阅读全文
相关推荐
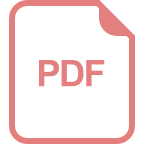
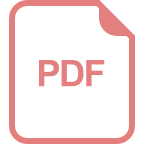
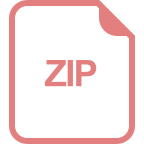

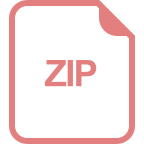
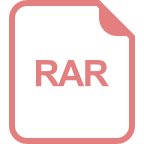
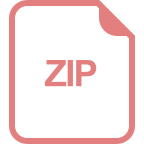
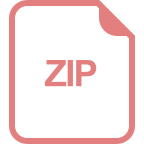
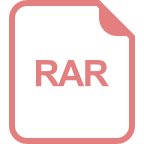
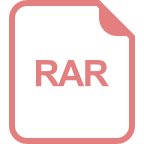
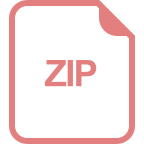

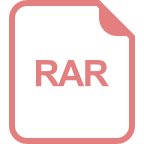
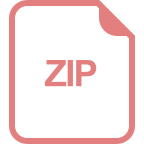