从.csv文件中读取用户(用户名、联系方式等)信息, 1.以用户名为关键字,创建二叉排序树存放信息 2.实现用户信息的查找 3.实现用户信息的新增
时间: 2024-04-07 14:33:07 浏览: 16
好的,我明白了。让我来回答你的问题。
首先,你可以使用Python中的pandas库来读取.csv文件中的用户信息。这里假设你的.csv文件中有"用户名"和"联系方式"两列。
1. 以用户名为关键字,创建二叉排序树存放信息:
首先,你需要定义一个二叉树节点的类,包含用户名、联系方式和左右子节点的引用。然后,遍历读取的每一行数据,创建节点并将其插入二叉排序树中。根据二叉排序树的特性,左子节点的值应小于父节点的值,右子节点的值应大于父节点的值。
以下是示例代码:
```python
import pandas as pd
class TreeNode:
def __init__(self, username, contact):
self.username = username
self.contact = contact
self.left = None
self.right = None
def insert_node(root, username, contact):
if root is None:
return TreeNode(username, contact)
if username < root.username:
root.left = insert_node(root.left, username, contact)
elif username > root.username:
root.right = insert_node(root.right, username, contact)
return root
# 读取.csv文件
data = pd.read_csv('user_info.csv')
root = None
for index, row in data.iterrows():
username = row['用户名']
contact = row['联系方式']
root = insert_node(root, username, contact)
```
2. 实现用户信息的查找:
为了查找用户信息,你可以定义一个递归函数来搜索二叉排序树。根据给定的用户名,比较它与当前节点的值,然后根据比较结果选择往左子树或右子树搜索,直到找到匹配的节点或遍历完整个树。
以下是示例代码:
```python
def search_user(root, username):
if root is None or root.username == username:
return root
if username < root.username:
return search_user(root.left, username)
else:
return search_user(root.right, username)
# 搜索用户信息
target_username = 'John'
target_node = search_user(root, target_username)
if target_node is not None:
print("联系方式:", target_node.contact)
else:
print("用户不存在")
```
3. 实现用户信息的新增:
新增用户信息只需在二叉排序树中插入一个新节点。根据用户名的大小关系,找到合适的位置插入节点。
以下是示例代码:
```python
def insert_user(root, username, contact):
if root is None:
return TreeNode(username, contact)
if username < root.username:
root.left = insert_user(root.left, username, contact)
elif username > root.username:
root.right = insert_user(root.right, username, contact)
return root
# 新增用户信息
new_username = 'Alice'
new_contact = '123456789'
root = insert_user(root, new_username, new_contact)
```
以上是回答你的问题的代码示例。希望能对你有所帮助!如果还有其他问题,请随时提问。
相关推荐
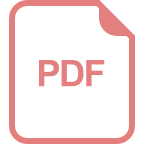
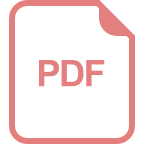














