C语言utf8转unicode代码
时间: 2023-07-27 14:07:06 浏览: 69
以下是一个简单的C语言函数,用于将UTF-8编码的字符转换为Unicode编码:
```
#include <stdio.h>
#include <string.h>
// 将UTF-8编码的字符转换为Unicode编码
int utf8_to_unicode(const char* utf8_str, int* unicode)
{
int len = strlen(utf8_str);
int i = 0, j = 0;
unsigned char byte1, byte2, byte3;
while (i < len) {
byte1 = utf8_str[i++];
if ((byte1 & 0x80) == 0) {
// 单字节字符
unicode[j++] = byte1;
} else if ((byte1 & 0xE0) == 0xC0) {
// 双字节字符
byte2 = utf8_str[i++];
unicode[j++] = ((byte1 & 0x1F) << 6) | (byte2 & 0x3F);
} else if ((byte1 & 0xF0) == 0xE0) {
// 三字节字符
byte2 = utf8_str[i++];
byte3 = utf8_str[i++];
unicode[j++] = ((byte1 & 0x0F) << 12) | ((byte2 & 0x3F) << 6) | (byte3 & 0x3F);
} else {
// 不支持的字符编码
return -1;
}
}
return j;
}
```
该函数的参数`utf8_str`是一个指向UTF-8编码字符串的指针,`unicode`是一个用于存储Unicode编码的整型数组。
函数返回转换后的Unicode字符数。如果遇到不支持的字符编码,则返回-1。
以下是一个示例程序,演示了如何使用该函数:
```
#include <stdio.h>
int utf8_to_unicode(const char* utf8_str, int* unicode);
int main()
{
const char* utf8_str = "Hello, 世界!";
int unicode[100];
int len = utf8_to_unicode(utf8_str, unicode);
if (len == -1) {
printf("不支持的字符编码\n");
return -1;
}
printf("UTF-8编码:%s\n", utf8_str);
printf("Unicode编码:");
for (int i = 0; i < len; i++) {
printf("%04X ", unicode[i]);
}
printf("\n");
return 0;
}
```
该程序将输出以下内容:
```
UTF-8编码:Hello, 世界!
Unicode编码:0048 0065 006C 006C 006F 002C 4E16 754C 0021
```
其中,Unicode编码对应的是字符串`"Hello, 世界!"`中每个字符的Unicode编码。
相关推荐
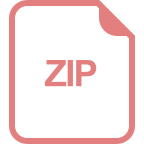














