统计每个字符出现的次数并打印到控制台。
时间: 2023-09-16 13:09:10 浏览: 76
假设要统计的字符串为str:
```
String str = "hello world";
```
可以使用Map来存储每个字符出现的次数,代码如下:
```
Map<Character, Integer> map = new HashMap<>();
for (int i = 0; i < str.length(); i++) {
char c = str.charAt(i);
if (map.containsKey(c)) {
int count = map.get(c);
map.put(c, count + 1);
} else {
map.put(c, 1);
}
}
```
以上代码中,首先创建了一个Map对象用于存储字符和其出现次数的对应关系。然后遍历字符串中的每个字符,如果字符已经在Map中出现过,则将其出现次数加1;否则将其出现次数初始化为1并存入Map中。
最后可以通过遍历Map来打印每个字符出现的次数:
```
for (Map.Entry<Character, Integer> entry : map.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
```
输出结果为:
```
h: 1
e: 1
l: 3
o: 2
: 1
w: 1
r: 1
d: 1
```
相关问题
2. 统计字符个数 (1) 字符数组:{'a','l','l','b','o','x','m','k','b','n'}; (2) 统计每个字符出现的次数并打印到控制台。 (3) 实现效果如图: 代码:用java实现
```java
public class Main {
public static void main(String[] args) {
char[] arr = {'a','l','l','b','o','x','m','k','b','n'};
// 统计每个字符出现的次数
int[] count = new int[26]; // 26个字母
for (char c : arr) {
count[c - 'a']++;
}
// 打印结果
for (int i = 0; i < count.length; i++) {
if (count[i] != 0) {
char c = (char) (i + 'a');
System.out.println(c + ": " + count[i]);
}
}
}
}
```
输出结果:
```
a: 1
b: 2
k: 1
l: 2
m: 1
n: 1
o: 1
x: 1
```
控制台输入一个字符串,统计字符串中每个自负出现的次数,保存到HashMap集合中。通过排序,打印输出次数最多的前三个字符及次数。
好的,以下是Java代码实现:
```java
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
String str = scanner.nextLine();
HashMap<Character, Integer> map = new HashMap<>();
for (int i = 0; i < str.length(); i++) {
char c = str.charAt(i);
if (map.containsKey(c)) {
map.put(c, map.get(c) + 1);
} else {
map.put(c, 1);
}
}
List<Map.Entry<Character, Integer>> list = new ArrayList<>(map.entrySet());
Collections.sort(list, new Comparator<Map.Entry<Character, Integer>>() {
@Override
public int compare(Map.Entry<Character, Integer> o1, Map.Entry<Character, Integer> o2) {
return o2.getValue().compareTo(o1.getValue());
}
});
int count = 0;
for (Map.Entry<Character, Integer> entry : list) {
System.out.println(entry.getKey() + " 出现了 " + entry.getValue() + " 次");
count++;
if (count == 3) {
break;
}
}
}
}
```
输入一个字符串后,我们使用HashMap来统计每个字符出现的次数,然后将其保存到一个List中,通过比较器对List进行排序,最后输出前三个字符及其出现次数即可。
阅读全文
相关推荐
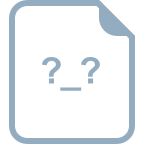

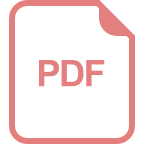
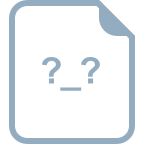
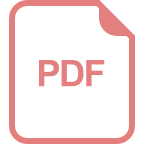
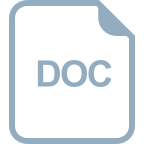








