设链表结点结构已经给出,试编写链表插入函数Insert将实现链表末尾添加数据,再编写函数Print,实现链表中链表数据打印,编写Destroy函数,实现链表的销毁释放,并在main函数中写出完整的调用过程。 typedef struct node { char Name[20]; struct node *Next; }Node;
时间: 2023-07-11 09:50:44 浏览: 88
好的,我可以根据你的需求回答这个问题。
首先,我们需要定义一个链表结点结构,结构体中包含一个字符串Name和一个指向下一个结点的指针Next。代码如下:
```
typedef struct node {
char Name[20];
struct node *Next;
} Node;
```
接下来,我们来实现链表插入函数Insert,代码如下:
```
void Insert(Node **head, char *name) {
Node *newNode = (Node*)malloc(sizeof(Node));
strcpy(newNode->Name, name);
newNode->Next = NULL;
if (*head == NULL) {
*head = newNode;
return;
}
Node *tmp = *head;
while (tmp->Next != NULL) {
tmp = tmp->Next;
}
tmp->Next = newNode;
}
```
这个Insert函数的作用是将一个新的结点添加到链表的末尾。
接下来,我们来实现链表打印函数Print,代码如下:
```
void Print(Node *head) {
Node *tmp = head;
while (tmp != NULL) {
printf("%s ", tmp->Name);
tmp = tmp->Next;
}
printf("\n");
}
```
这个Print函数的作用是将整个链表中的数据打印出来。
最后,我们来实现链表销毁函数Destroy,代码如下:
```
void Destroy(Node **head) {
Node *current = *head;
while (current != NULL) {
Node *next = current->Next;
free(current);
current = next;
}
*head = NULL;
}
```
这个Destroy函数的作用是将整个链表销毁并释放内存。
最后,我们在main函数中调用这些函数,代码如下:
```
int main() {
Node *head = NULL;
Insert(&head, "Alice");
Insert(&head, "Bob");
Insert(&head, "Charlie");
Print(head);
Destroy(&head);
return 0;
}
```
这个main函数的作用是创建一个链表,向链表中添加三个数据,然后打印链表中的数据,最后销毁整个链表并释放内存。
希望我的回答能够帮助你解决问题,如果有其他问题,请随时问我!
阅读全文
相关推荐
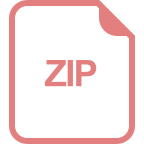
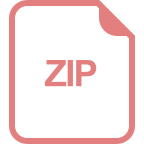
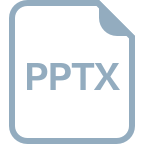
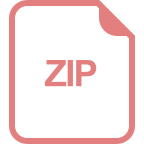
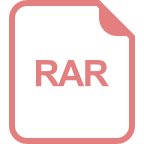
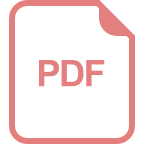
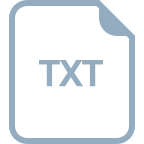
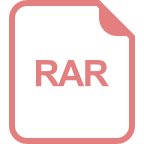
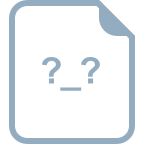
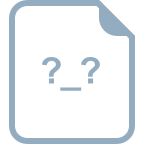
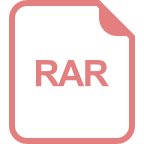
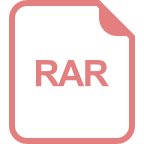