51单片机 按键矩阵 数码管 除法运算 小数点 编程
时间: 2024-03-11 08:48:52 浏览: 88
好的,为了更好的帮助你,我将会提供一个简单的51单片机程序代码,实现按键矩阵输入数字和操作符,进行小数点除法运算,最后在数码管上显示结果。代码如下:
```c
#include <reg51.h>
#define uchar unsigned char
#define uint unsigned int
sbit Key_1 = P2^0; // 按键矩阵第1行
sbit Key_2 = P2^1; // 按键矩阵第2行
sbit Key_3 = P2^2; // 按键矩阵第3行
sbit Key_4 = P2^3; // 按键矩阵第4行
sbit Key_5 = P2^4; // 按键矩阵第1列
sbit Key_6 = P2^5; // 按键矩阵第2列
sbit Key_7 = P2^6; // 按键矩阵第3列
sbit Key_8 = P2^7; // 按键矩阵第4列
sbit D1 = P1^0; // 数码管第1位
sbit D2 = P1^1; // 数码管第2位
sbit D3 = P1^2; // 数码管第3位
sbit D4 = P1^3; // 数码管第4位
uchar Key_Value; // 按键值
uchar Key_Flag; // 按键标志
uchar Key_Buffer[4]; // 按键缓存
uint Num1, Num2; // 操作数1、操作数2
uchar Operator; // 操作符
float Result; // 计算结果
// 数码管显示函数
void Display(float num) {
uint temp = 0;
uchar i, j;
uchar code table[] = { // 数码管段码表
0x3F, 0x06, 0x5B, 0x4F, 0x66,
0x6D, 0x7D, 0x07, 0x7F, 0x6F
};
temp = num * 1000; // 将小数转换为整数
for (i = 0; i < 4; i++) {
j = temp % 10; // 取个位数
switch (i) {
case 0: D1 = 1; D2 = 0; D3 = 0; D4 = 0; break;
case 1: D1 = 0; D2 = 1; D3 = 0; D4 = 0; break;
case 2: D1 = 0; D2 = 0; D3 = 1; D4 = 0; break;
case 3: D1 = 0; D2 = 0; D3 = 0; D4 = 1; break;
}
P0 = table[j]; // 显示数码管
delay(1); // 稍微延时
P0 = 0x00; // 关闭数码管
temp /= 10; // 取下一位数
}
}
// 获取按键值函数
uchar Get_Key(void) {
Key_1 = 0; Key_2 = 1; Key_3 = 1; Key_4 = 1;
if (Key_5 == 0) { delay(5); if (Key_5 == 0) return 1; }
if (Key_6 == 0) { delay(5); if (Key_6 == 0) return 2; }
if (Key_7 == 0) { delay(5); if (Key_7 == 0) return 3; }
if (Key_8 == 0) { delay(5); if (Key_8 == 0) return 10; }
Key_1 = 1; Key_2 = 0; Key_3 = 1; Key_4 = 1;
if (Key_5 == 0) { delay(5); if (Key_5 == 0) return 4; }
if (Key_6 == 0) { delay(5); if (Key_6 == 0) return 5; }
if (Key_7 == 0) { delay(5); if (Key_7 == 0) return 6; }
if (Key_8 == 0) { delay(5); if (Key_8 == 0) return 11; }
Key_1 = 1; Key_2 = 1; Key_3 = 0; Key_4 = 1;
if (Key_5 == 0) { delay(5); if (Key_5 == 0) return 7; }
if (Key_6 == 0) { delay(5); if (Key_6 == 0) return 8; }
if (Key_7 == 0) { delay(5); if (Key_7 == 0) return 9; }
if (Key_8 == 0) { delay(5); if (Key_8 == 0) return 12; }
Key_1 = 1; Key_2 = 1; Key_3 = 1; Key_4 = 0;
if (Key_5 == 0) { delay(5); if (Key_5 == 0) return 13; }
if (Key_6 == 0) { delay(5); if (Key_6 == 0) return 0; }
if (Key_7 == 0) { delay(5); if (Key_7 == 0) return 14; }
if (Key_8 == 0) { delay(5); if (Key_8 == 0) return 15; }
return 16;
}
// 获取按键函数
void Get_Key_Value(void) {
uchar i;
Key_Value = Get_Key();
if (Key_Value == 16) return;
if (Key_Value == 14 && !Key_Flag) return;
if (Key_Value == 13 && !Key_Flag) { // 清除
for (i = 0; i < 4; i++) Key_Buffer[i] = 0;
Num1 = 0; Num2 = 0; Operator = 0; Result = 0;
Display(0); // 将数码管清零
}
if (Key_Value == 14 && Key_Flag) { // 计算
Num2 = atoi(Key_Buffer);
Key_Flag = 0;
switch (Operator) {
case 11: Result = (float)Num1 / Num2; break; // 小数点除法
default: break;
}
Display(Result); // 显示结果
}
if (Key_Value >= 0 && Key_Value <= 9) { // 数字键
if (Key_Flag) {
for (i = 0; i < 3; i++) Key_Buffer[i] = Key_Buffer[i+1];
Key_Buffer[3] = Key_Value + '0';
Display(atof(Key_Buffer)); // 显示输入的数值
} else {
for (i = 0; i < 4; i++) Key_Buffer[i] = 0;
Key_Buffer[0] = Key_Value + '0';
Display(Key_Value); // 显示输入的数值
}
}
if (Key_Value >= 10 && Key_Value <= 12) { // 操作符
Operator = Key_Value;
Num1 = atoi(Key_Buffer);
Key_Flag = 1;
}
}
// 延时函数
void delay(uint t) {
uint i, j;
for (i = 0; i < t; i++)
for (j = 0; j < 120; j++);
}
// 主函数
void main() {
while (1) {
Get_Key_Value(); // 获取按键值
}
}
```
这是一个比较简单的程序,只实现了小数点除法运算,可以根据需要进行修改和扩展。希望我的回答对你有所帮助。
阅读全文
相关推荐
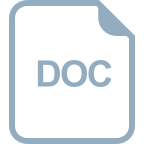
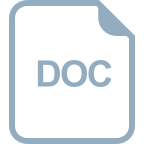
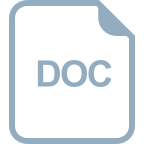
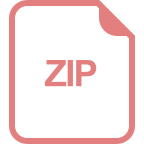
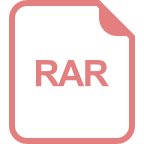
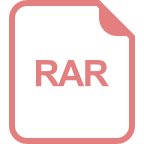
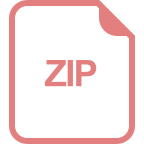
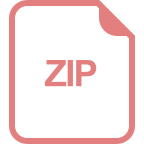
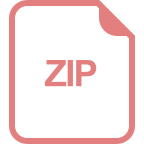
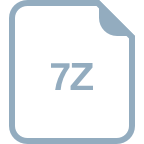
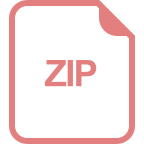
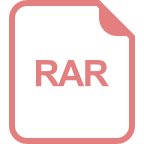
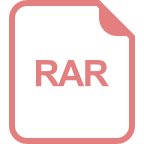
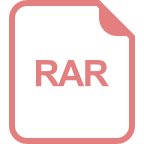
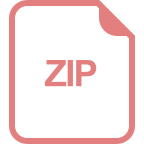
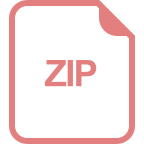
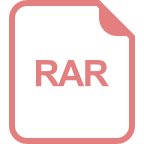
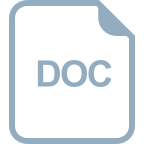
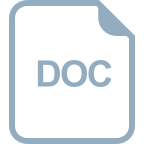