linux+arm怎么使用AudioPlay
时间: 2023-06-11 08:10:05 浏览: 155
在Linux ARM架构下,您可以使用ALSA(Advanced Linux Sound Architecture)来播放音频。以下是使用ALSA库进行音频播放的基本步骤:
1. 安装ALSA库:在终端中使用以下命令安装ALSA库:
```
sudo apt-get install libasound2-dev
```
2. 编写代码:您需要使用ALSA库的函数来设置音频参数,打开音频设备,并将音频数据写入设备缓冲区。以下是一个简单的示例代码,用于播放WAV格式的音频文件:
```
#include <stdio.h>
#include <stdlib.h>
#include <alsa/asoundlib.h>
#define BUFFER_SIZE 4096
int main(int argc, char *argv[])
{
int err;
char *buffer;
snd_pcm_t *handle;
snd_pcm_hw_params_t *params;
snd_pcm_uframes_t frames;
int channels, rate, bits;
long unsigned int buffer_size;
if (argc != 2) {
printf("Usage: %s <file>\n", argv[0]);
return 1;
}
FILE *fp = fopen(argv[1], "rb");
if (fp == NULL) {
printf("Error: Failed to open file %s\n", argv[1]);
return 1;
}
// Get the WAV file parameters
fseek(fp, 22, SEEK_SET);
fread(&channels, 2, 1, fp);
fseek(fp, 24, SEEK_SET);
fread(&rate, 4, 1, fp);
fseek(fp, 34, SEEK_SET);
fread(&bits, 2, 1, fp);
// Open the audio device for playback
err = snd_pcm_open(&handle, "default", SND_PCM_STREAM_PLAYBACK, 0);
if (err < 0) {
printf("Error: Failed to open audio device\n");
return 1;
}
// Allocate the hardware parameters object
err = snd_pcm_hw_params_malloc(¶ms);
if (err < 0) {
printf("Error: Failed to allocate hardware parameters object\n");
return 1;
}
// Initialize the hardware parameters object with default values
err = snd_pcm_hw_params_any(handle, params);
if (err < 0) {
printf("Error: Failed to initialize hardware parameters object\n");
return 1;
}
// Set the desired hardware parameters
err = snd_pcm_hw_params_set_access(handle, params, SND_PCM_ACCESS_RW_INTERLEAVED);
if (err < 0) {
printf("Error: Failed to set access type\n");
return 1;
}
err = snd_pcm_hw_params_set_format(handle, params, SND_PCM_FORMAT_S16_LE);
if (err < 0) {
printf("Error: Failed to set sample format\n");
return 1;
}
err = snd_pcm_hw_params_set_channels(handle, params, channels);
if (err < 0) {
printf("Error: Failed to set channel count\n");
return 1;
}
err = snd_pcm_hw_params_set_rate_near(handle, params, &rate, 0);
if (err < 0) {
printf("Error: Failed to set sample rate\n");
return 1;
}
// Write the hardware parameters to the audio device
err = snd_pcm_hw_params(handle, params);
if (err < 0) {
printf("Error: Failed to set hardware parameters\n");
return 1;
}
// Calculate the buffer size in frames
snd_pcm_hw_params_get_period_size(params, &frames, 0);
buffer_size = frames * channels * bits / 8;
// Allocate the buffer for audio data
buffer = (char *)malloc(buffer_size);
if (buffer == NULL) {
printf("Error: Failed to allocate buffer for audio data\n");
return 1;
}
// Read the WAV file data into the buffer and write it to the audio device
while (fread(buffer, buffer_size, 1, fp) == 1) {
err = snd_pcm_writei(handle, buffer, frames);
if (err < 0) {
printf("Error: Failed to write audio data to device\n");
return 1;
}
}
// Close the audio device and free resources
snd_pcm_drain(handle);
snd_pcm_close(handle);
free(buffer);
fclose(fp);
return 0;
}
```
3. 编译代码:在终端中使用以下命令编译代码:
```
gcc -o audioplay audioplay.c -lasound
```
4. 运行代码:在终端中使用以下命令运行代码,并将WAV格式的音频文件作为参数传递给程序:
```
./audioplay <file>
```
其中,`<file>`为WAV格式的音频文件路径。
阅读全文
相关推荐
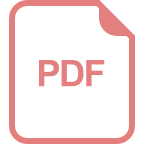
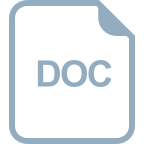
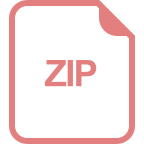















