c++编写一个插入的代码用于信息添加
时间: 2024-10-18 12:06:33 浏览: 14
在C++中,如果你想创建一个简单的程序来插入信息,你可以使用一个容器,如`std::vector`或`std::list`。这里是一个基本的例子,展示如何使用`std::vector`来存储一些简单的键值对,并允许用户插入新的信息:
```cpp
#include <iostream>
#include <vector>
#include <string>
// 创建一个简单的结构体表示信息
struct Information {
std::string key;
std::string value;
};
// 插入函数
void insertInformation(std::vector<Information>& informations, const std::string& key, const std::string& value) {
Information newInfo = {key, value};
informations.push_back(newInfo);
}
int main() {
std::vector<Information> myInformations;
// 提示用户输入并插入信息
std::cout << "请输入键值对 (例如 'name John Doe'),输入'quit'结束:\n";
while (true) {
std::string input;
std::getline(std::cin, input);
if (input == "quit") break;
size_t pos = input.find(' ');
if (pos != std::string::npos) {
insertInformation(myInformations, input.substr(0, pos), input.substr(pos + 1));
} else {
std::cerr << "无效的输入,格式应为 'key value'" << std::endl;
}
}
// 打印所有信息
for (const auto& info : myInformations) {
std::cout << "Key: " << info.key << ", Value: " << info.value << std::endl;
}
return 0;
}
```
在这个例子中,用户可以输入键值对,程序会将其存储到`myInformations`向量中。当你运行这个程序并想要停止输入时,只需输入"quit"。
阅读全文
相关推荐
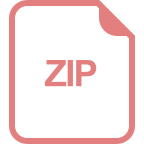
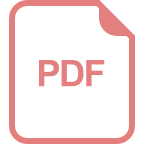
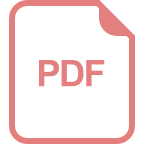
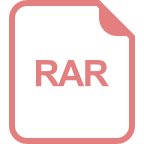
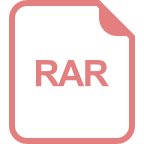
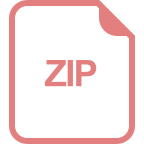
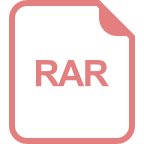
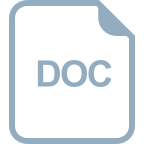
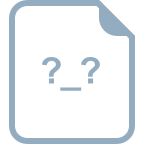
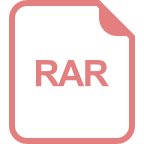
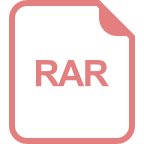
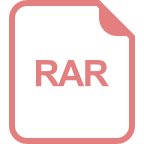
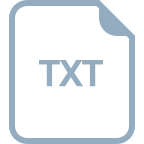
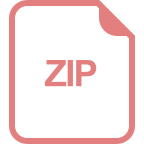
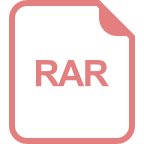
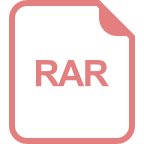
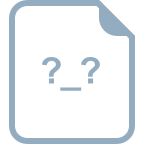