c语言对于填写不同的四个专业按专业优先录取(从第一个开始)有关代码举例
时间: 2023-08-15 13:05:23 浏览: 48
以下是一个简单的 C 语言示例代码,用于按照专业优先录取学生。这里假设有四个专业:计算机科学、电子工程、机械工程和化学工程,并且学生的信息已经存储在一个结构体数组中,每个结构体包含姓名、专业和成绩等信息。代码如下:
```c
#include <stdio.h>
#include <string.h>
typedef struct {
char name[20];
char major[20];
int score;
} Student;
int main() {
Student students[4] = {
{"Tom", "Computer Science", 85},
{"Jack", "Electronic Engineering", 78},
{"Lucy", "Mechanical Engineering", 92},
{"Lily", "Chemical Engineering", 80}
};
char majors[4][20] = {
"Computer Science",
"Electronic Engineering",
"Mechanical Engineering",
"Chemical Engineering"
};
// 冒泡排序,按专业优先级排序
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3 - i; j++) {
int m1 = -1, m2 = -1;
for (int k = 0; k < 4; k++) {
if (strcmp(students[k].major, majors[j]) == 0) {
m1 = k;
}
if (strcmp(students[k].major, majors[j+1]) == 0) {
m2 = k;
}
}
if (m1 != -1 && m2 != -1 && students[m1].score < students[m2].score) {
Student temp = students[m1];
students[m1] = students[m2];
students[m2] = temp;
}
}
}
// 输出排序后的结果
printf("按专业优先级排序后的录取结果:\n");
for (int i = 0; i < 4; i++) {
printf("%s\t%s\t%d\n", students[i].name, students[i].major, students[i].score);
}
return 0;
}
```
在这个示例代码中,我们首先定义了一个 `Student` 结构体,用于存储学生信息。然后定义了一个结构体数组 `students`,包含了四个学生的信息。接着定义了一个字符串数组 `majors`,用于存储专业名称,按照优先级从高到低排列。接下来,我们使用冒泡排序算法对学生信息进行排序,排序的依据是专业优先级和成绩。最后输出排序后的结果。
相关推荐
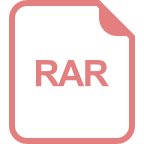
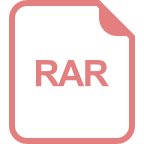
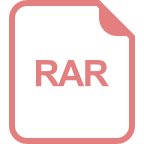














