vue3中,使用three.js建立一个3D农田
时间: 2023-07-21 22:00:23 浏览: 201
在Vue 3中使用Three.js建立一个3D农田,你可以按照以下步骤进行:
1. 首先,确保你已经安装了Vue 3和Three.js。你可以使用Vue CLI来创建一个新的Vue项目,然后使用npm或yarn安装Three.js。
2. 在Vue项目的组件中,引入Three.js库。你可以在组件的`<script>`标签中使用`import * as THREE from 'three'`来引入Three.js。
3. 在组件的`mounted`生命周期钩子中,创建一个场景(Scene)、相机(Camera)和渲染器(Renderer)。例如:
```javascript
mounted() {
// 创建场景
this.scene = new THREE.Scene();
// 创建相机
this.camera = new THREE.PerspectiveCamera(
75,
window.innerWidth / window.innerHeight,
0.1,
1000
);
this.camera.position.z = 5;
// 创建渲染器
this.renderer = new THREE.WebGLRenderer({ antialias: true });
this.renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(this.renderer.domElement);
}
```
4. 在组件中创建农田的模型。你可以使用Three.js的几何体(Geometry)和材质(Material)来创建不同的物体。例如,你可以使用`BoxGeometry`来创建一个方块作为农田的地块,然后使用`MeshBasicMaterial`来给地块上色。例如:
```javascript
mounted() {
// ...
// 创建地块模型
const geometry = new THREE.BoxGeometry(1, 1, 1);
const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 });
this.field = new THREE.Mesh(geometry, material);
this.scene.add(this.field);
}
```
5. 添加光源。在Three.js中,光源是非常重要的,它可以影响物体的阴影和反射等效果。你可以使用`AmbientLight`来添加一个环境光,以及`PointLight`或`DirectionalLight`来添加其他类型的光源。例如:
```javascript
mounted() {
// ...
// 添加环境光
const ambientLight = new THREE.AmbientLight(0x404040);
this.scene.add(ambientLight);
// 添加平行光
const directionalLight = new THREE.DirectionalLight(0xffffff);
directionalLight.position.set(1, 1, 1).normalize();
this.scene.add(directionalLight);
}
```
6. 在Vue组件中添加动画循环。你可以使用Vue的`requestAnimationFrame`来实现动画循环,并在每一帧更新场景的状态。例如:
```javascript
mounted() {
// ...
this.animate();
},
methods: {
animate() {
requestAnimationFrame(this.animate);
// 在这里更新场景状态,例如旋转地块
this.field.rotation.x += 0.01;
this.field.rotation.y += 0.01;
this.renderer.render(this.scene, this.camera);
}
}
```
这样,你就可以在Vue 3中使用Three.js建立一个简单的3D农田了。当然,这只是一个基本的示例,你可以根据自己的需求来扩展和美化场景。
阅读全文
相关推荐
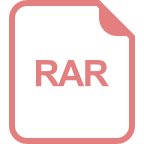
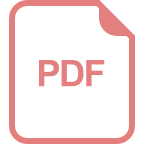
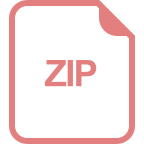
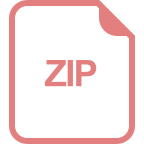
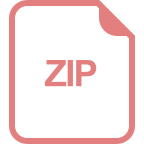
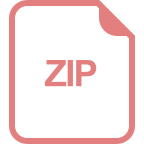
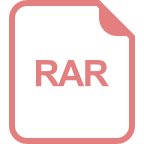
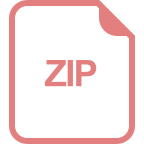
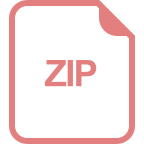