python提取页面链接
时间: 2023-06-12 14:03:41 浏览: 162
可以使用Python的requests和BeautifulSoup库提取页面链接。以下是一个简单的示例代码:
```python
import requests
from bs4 import BeautifulSoup
url = "https://www.example.com"
response = requests.get(url)
soup = BeautifulSoup(response.text, "html.parser")
links = []
for link in soup.find_all("a"):
href = link.get("href")
if href:
links.append(href)
print(links)
```
该代码通过requests库获取网页内容,然后使用BeautifulSoup库解析HTML,并使用find_all方法查找所有<a>标签。对于每个<a>标签,我们获取其href属性并将其添加到链接列表中。最后,我们打印链接列表。
相关问题
写一段python代码提取页面数据
以下是提取页面数据的 Python 代码示例:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://www.example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# 提取页面标题
title = soup.title.string
print('页面标题:', title)
# 提取页面所有链接
links = []
for link in soup.find_all('a'):
links.append(link.get('href'))
print('页面链接:', links)
# 提取页面所有图片链接
img_links = []
for img in soup.find_all('img'):
img_links.append(img.get('src'))
print('页面图片链接:', img_links)
```
以上代码使用 requests 库获取页面内容,使用 BeautifulSoup 库解析页面,然后提取页面标题、链接和图片链接。
python提取xpath内容
在Python中使用XPath提取网页内容通常涉及以下几个步骤:
1. 安装必要的库[^2]:
```python
!pip install lxml
```
或者选择其他如BeautifulSoup。
2. 导入所需的库并解析HTML文档:
```python
from bs4 import BeautifulSoup
import requests
url = "你的网页URL"
response = requests.get(url)
soup = BeautifulSoup(response.text, 'lxml')
```
3. 使用`lxml`库的`ElementTree`模块执行XPath查询:
```python
# 使用XPath表达式提取标题元素
names_xpath = '//h4/a/text()'
titles = soup.xpath(names_xpath)
titles_list = [title.strip() for title in titles] # 去除空白字符
```
这里,`//h4/a/text()`表示查找所有`<a>`标签位于`<h4>`标签下的文本。
4. 打印结果:
```python
print(titles_list)
```
注意,XPath表达式的具体写法会因网页结构不同而变化,上述例子假设网页有`<h4>`标签包裹着链接,链接文字作为标题。如果实际页面结构不同,可能需要调整XPath路径。
阅读全文
相关推荐
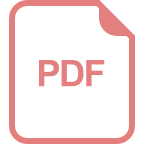
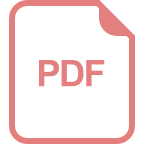

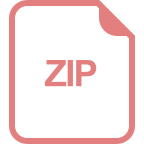
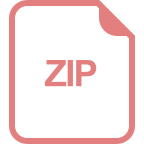
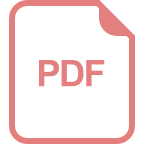
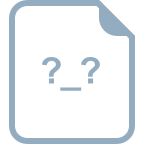
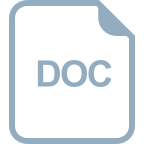
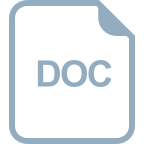



