如果在for-else语句中不用break会怎么执行代码,请举例
时间: 2024-05-14 15:19:56 浏览: 13
在 Python 中,for-else 语句中的 else 子句是在循环正常结束后执行的,如果循环中没有出现 break 语句则会执行 else 子句。如果在循环中使用了 break 语句,则 else 子句不会执行。
以下是一个示例,演示了 for-else 语句中不使用 break 时的执行情况:
```python
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
if fruit == "orange":
print("I found an orange!")
break
else:
print("I did not find any oranges")
```
在这个示例中,我们遍历了一个水果列表。如果找到了一个橙子,我们将打印一条消息并使用 break 语句退出循环。否则,我们会在循环正常结束时执行 else 子句,并打印一条消息。
假设我们没有在列表中找到橙子,那么输出结果将是:
```
I did not find any oranges
```
这是因为循环正常结束后会执行 else 子句。如果我们将列表中的某个水果更改为 "orange",则输出结果将是:
```
I found an orange!
```
因为我们找到了一个橙子并使用了 break 语句,因此 else 子句不会执行。
相关问题
python的for循环如何在特定条件下跳出,请举例说明
在Python中,可以使用break语句来在特定条件下跳出for循环。例如,假设我们要在列表中查找特定的元素,并在找到该元素后退出循环:
```
my_list = [1, 2, 3, 4, 5]
for num in my_list:
if num == 3:
print("Found the number 3!")
break
else:
print(num)
```
在这个例子中,我们使用for循环遍历列表my_list中的元素。当找到元素3时,我们使用break语句退出循环。如果没有找到元素3,for循环将继续执行并打印列表中的其他元素。
举例说明怎么改善java中多if的语句
假设有如下代码:
```java
if (x == 1) {
// do something
} else if (x == 2) {
// do something else
} else if (x == 3) {
// do something different
} else {
// default action
}
```
可以通过以下方法改善:
1. 使用switch语句代替if-else语句
```java
switch (x) {
case 1:
// do something
break;
case 2:
// do something else
break;
case 3:
// do something different
break;
default:
// default action
break;
}
```
2. 使用策略模式
```java
interface Action {
void doAction();
}
class ActionOne implements Action {
@Override
public void doAction() {
// do something
}
}
class ActionTwo implements Action {
@Override
public void doAction() {
// do something else
}
}
class ActionThree implements Action {
@Override
public void doAction() {
// do something different
}
}
Map<Integer, Action> actionMap = new HashMap<>();
actionMap.put(1, new ActionOne());
actionMap.put(2, new ActionTwo());
actionMap.put(3, new ActionThree());
if (actionMap.containsKey(x)) {
actionMap.get(x).doAction();
} else {
// default action
}
```
3. 使用工厂模式
```java
interface Action {
void doAction();
}
class ActionOne implements Action {
@Override
public void doAction() {
// do something
}
}
class ActionTwo implements Action {
@Override
public void doAction() {
// do something else
}
}
class ActionThree implements Action {
@Override
public void doAction() {
// do something different
}
}
class ActionFactory {
public static Action getAction(int x) {
switch (x) {
case 1:
return new ActionOne();
case 2:
return new ActionTwo();
case 3:
return new ActionThree();
default:
return null;
}
}
}
Action action = ActionFactory.getAction(x);
if (action != null) {
action.doAction();
} else {
// default action
}
```
相关推荐
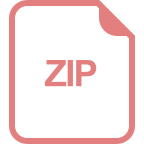
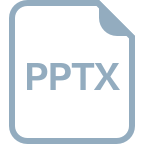
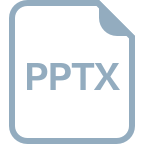











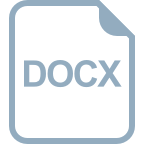
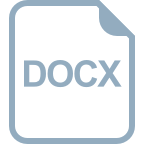