我现在需要处理微博内容的文本,其中有,#()等等,有已经被人写好的处理微博内容的文本分析预处理代码嘛
时间: 2024-03-14 16:45:09 浏览: 28
是的,处理微博内容的文本预处理代码有很多,以下是一些常用的预处理步骤和代码示例:
1. 去除HTML标签和URL链接
```python
import re
def clean_html(text):
"""去除HTML标签"""
html_pattern = re.compile('<.*?>')
return html_pattern.sub('', text)
def clean_url(text):
"""去除URL链接"""
url_pattern = re.compile(r'http\S+')
return url_pattern.sub('', text)
```
2. 去除@用户和#话题
```python
def clean_at(text):
"""去除@用户"""
at_pattern = re.compile(r'@[^\s]+')
return at_pattern.sub('', text)
def clean_topic(text):
"""去除#话题"""
topic_pattern = re.compile(r'#[^#]+#')
return topic_pattern.sub('', text)
```
3. 去除表情符号和特殊字符
```python
def clean_emoji(text):
"""去除表情符号"""
emoji_pattern = re.compile('[\U00010000-\U0010ffff]', flags=re.UNICODE)
return emoji_pattern.sub('', text)
def clean_special_char(text):
"""去除特殊字符"""
special_char_pattern = re.compile('[^A-Za-z0-9\u4e00-\u9fa5\ ]+')
return special_char_pattern.sub('', text)
```
4. 分词和去除停用词
```python
import jieba
import jieba.analyse
import pandas as pd
# 加载停用词
stopwords = pd.read_csv('stopwords.txt', index_col=False, quoting=3, sep="\t", names=['stopword'], encoding='utf-8')
stopwords = stopwords['stopword'].values
def cut_text(text):
"""分词"""
seg_list = jieba.cut(text)
return " ".join(seg_list)
def clean_stopwords(text):
"""去除停用词"""
words = text.split()
filtered_words = [word for word in words if word not in stopwords]
return " ".join(filtered_words)
```
将上述预处理步骤组合起来,可以得到一个完整的微博内容文本预处理函数:
```python
def clean_text(text):
"""微博内容文本预处理"""
text = clean_html(text) # 去除HTML标签
text = clean_url(text) # 去除URL链接
text = clean_at(text) # 去除@用户
text = clean_topic(text) # 去除#话题
text = clean_emoji(text) # 去除表情符号
text = clean_special_char(text) # 去除特殊字符
text = cut_text(text) # 分词
text = clean_stopwords(text) # 去除停用词
return text
```
这样,就可以将微博内容文本进行预处理,以便进行后续分析。
相关推荐
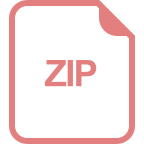
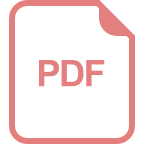
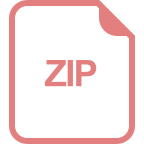











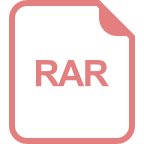
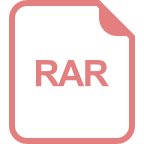
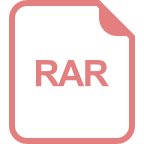
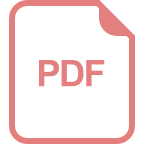