RTI实现通信示例C++
时间: 2023-10-20 09:23:57 浏览: 210
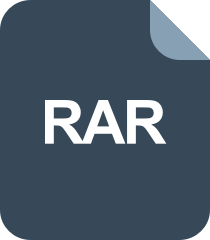
C++一个通信的实例
以下是一个简单的 RTI 通信示例,使用 C++ 编写。
在此示例中,我们将创建两个简单的应用程序:发布者和订阅者。发布者将发送一条简单的消息,而订阅者将接收该消息并将其打印到控制台。
首先,我们需要定义消息类型。在本例中,我们将使用一个名为 "HelloWorld" 的字符串消息:
```cpp
#include <string>
struct HelloWorld {
std::string message;
};
```
接下来,我们将使用 RTI Connext API 创建发布者和订阅者。
发布者代码:
```cpp
#include <ndds/ndds_cpp.h>
int main() {
// 创建域
DDSDomainParticipant *participant = DDSDomainParticipantFactory::get_instance()->create_participant(0, DDS_PARTICIPANT_QOS_DEFAULT, NULL, DDS_STATUS_MASK_NONE);
if (participant == NULL) {
// 错误处理
}
// 创建类型
HelloWorldTypeSupport *ts = new HelloWorldTypeSupport();
if (ts->register_type(participant, "") != DDS_RETCODE_OK) {
// 错误处理
}
// 创建主题
DDSTopic *topic = participant->create_topic("HelloWorldTopic", ts->get_type_name(), DDS_TOPIC_QOS_DEFAULT, NULL, DDS_STATUS_MASK_NONE);
if (topic == NULL) {
// 错误处理
}
// 创建发布者
DDSPublisher *publisher = participant->create_publisher(DDS_PUBLISHER_QOS_DEFAULT, NULL, DDS_STATUS_MASK_NONE);
if (publisher == NULL) {
// 错误处理
}
// 创建数据写入器
DDSDataWriter *writer = publisher->create_datawriter(topic, DDS_DATAWRITER_QOS_DEFAULT, NULL, DDS_STATUS_MASK_NONE);
if (writer == NULL) {
// 错误处理
}
// 发送消息
HelloWorldDataWriter *hw_writer = HelloWorldDataWriter::narrow(writer);
HelloWorld hello;
hello.message = "Hello, World!";
hw_writer->write(hello, DDS_HANDLE_NIL);
// 清理资源
participant->delete_contained_entities();
DDSDomainParticipantFactory::get_instance()->delete_participant(participant);
return 0;
}
```
订阅者代码:
```cpp
#include <ndds/ndds_cpp.h>
class HelloWorldListener : public DDSDataReaderListener {
public:
virtual void on_data_available(DDSDataReader *reader) {
HelloWorldDataReader *hw_reader = HelloWorldDataReader::narrow(reader);
HelloWorldSeq data_seq;
DDS_SampleInfoSeq info_seq;
DDS_ReturnCode_t retcode = hw_reader->take(data_seq, info_seq, DDS_LENGTH_UNLIMITED, DDS_ANY_SAMPLE_STATE, DDS_ANY_VIEW_STATE, DDS_ANY_INSTANCE_STATE);
if (retcode == DDS_RETCODE_NO_DATA) {
return;
} else if (retcode != DDS_RETCODE_OK) {
// 错误处理
}
for (int i = 0; i < data_seq.length(); i++) {
if (info_seq[i].valid_data) {
std::cout << "Received message: " << data_seq[i].message << std::endl;
}
}
hw_reader->return_loan(data_seq, info_seq);
}
};
int main() {
// 创建域
DDSDomainParticipant *participant = DDSDomainParticipantFactory::get_instance()->create_participant(0, DDS_PARTICIPANT_QOS_DEFAULT, NULL, DDS_STATUS_MASK_NONE);
if (participant == NULL) {
// 错误处理
}
// 创建类型
HelloWorldTypeSupport *ts = new HelloWorldTypeSupport();
if (ts->register_type(participant, "") != DDS_RETCODE_OK) {
// 错误处理
}
// 创建主题
DDSTopic *topic = participant->create_topic("HelloWorldTopic", ts->get_type_name(), DDS_TOPIC_QOS_DEFAULT, NULL, DDS_STATUS_MASK_NONE);
if (topic == NULL) {
// 错误处理
}
// 创建订阅者
DDSSubscriber *subscriber = participant->create_subscriber(DDS_SUBSCRIBER_QOS_DEFAULT, NULL, DDS_STATUS_MASK_NONE);
if (subscriber == NULL) {
// 错误处理
}
// 创建数据阅读器
DDSDataReader *reader = subscriber->create_datareader(topic, DDS_DATAREADER_QOS_DEFAULT, new HelloWorldListener(), DDS_STATUS_MASK_ALL);
if (reader == NULL) {
// 错误处理
}
// 进入主循环
while (true) {
// 可以在这里执行其他任务
NDDSUtility::sleep(dds::core::Duration::from_millisecs(100));
}
// 清理资源
participant->delete_contained_entities();
DDSDomainParticipantFactory::get_instance()->delete_participant(participant);
return 0;
}
```
注意,上述示例仅提供了基本的 RTI Connext API 使用方法。在实际应用程序中,您需要对发布者和订阅者进行更详细的配置和错误处理。
阅读全文
相关推荐
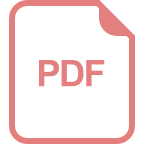
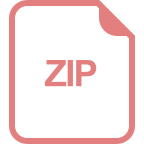
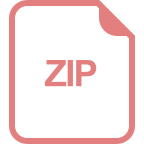
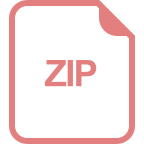
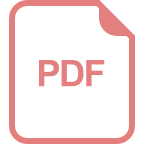

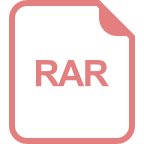
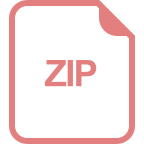
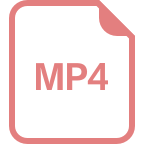
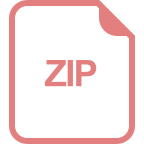