将下列代码转换成在mysql里创建表的格式class Post(models.Model): id = models.UUIDField(primary_key=True, default=uuid.uuid4) # 用户名 user = models.CharField(max_length=100) # 发的帖子图片 image = models.ImageField(upload_to='post_images') # 标题 caption = models.TextField() created_at = models.DateTimeField(default=datetime.now) # 点赞数 no_of_likes = models.IntegerField(default=0)
时间: 2024-03-02 20:52:47 浏览: 169
以下是将该代码转换成在 MySQL 中创建表格的格式:
```sql
CREATE TABLE Post (
id CHAR(32) NOT NULL PRIMARY KEY,
user VARCHAR(100) NOT NULL,
image VARCHAR(100) NOT NULL,
caption TEXT NOT NULL,
created_at DATETIME NOT NULL DEFAULT CURRENT_TIMESTAMP,
no_of_likes INT DEFAULT 0
);
```
注意,这是一个基本的表格结构,可能需要根据实际需求进行调整。例如,在MySQL中,可以使用不同的数据类型来存储图像,例如BLOB或LONGBLOB。此外,还需要为表格定义适当的索引和约束来确保数据的完整性和查询性能。
相关问题
学生成绩mvc mysql_大作业练习:用Asp.net Mvc4做一个:学生考试成绩管理系统-简易版...
好的,下面是一个简单的学生考试成绩管理系统的实现:
1. 创建数据库
首先在 MySQL 中创建一个名为 `student_score` 的数据库,然后创建以下两个表:
- `students` 表,用于存储学生信息,包括学生 ID、姓名和班级。
```
CREATE TABLE `students` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(50) DEFAULT NULL,
`class` varchar(50) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
```
- `scores` 表,用于存储学生考试成绩,包括学生 ID、科目和成绩。
```
CREATE TABLE `scores` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`student_id` int(11) DEFAULT NULL,
`subject` varchar(50) DEFAULT NULL,
`score` int(11) DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `FK_scores_students` (`student_id`),
CONSTRAINT `FK_scores_students` FOREIGN KEY (`student_id`) REFERENCES `students` (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
```
2. 创建 ASP.NET MVC4 项目
在 Visual Studio 中创建一个 ASP.NET MVC4 项目,选择 Empty 模板。
3. 添加模型
在项目中添加两个模型类 `Student` 和 `Score`,代码如下:
```
public class Student
{
public int Id { get; set; }
public string Name { get; set; }
public string Class { get; set; }
}
public class Score
{
public int Id { get; set; }
public int StudentId { get; set; }
public string Subject { get; set; }
public int Score { get; set; }
}
```
4. 添加控制器
在 Controllers 文件夹中添加一个名为 `HomeController` 的控制器,其中包含以下几个动作方法:
- `Index` 方法,用于显示学生列表和成绩列表。
```
public ActionResult Index()
{
var students = db.Students.ToList();
var scores = db.Scores.ToList();
ViewBag.Students = students;
ViewBag.Scores = scores;
return View();
}
```
- `AddStudent` 方法,用于添加新的学生信息。
```
[HttpPost]
public ActionResult AddStudent(Student student)
{
db.Students.Add(student);
db.SaveChanges();
return RedirectToAction("Index");
}
```
- `AddScore` 方法,用于添加新的成绩信息。
```
[HttpPost]
public ActionResult AddScore(Score score)
{
db.Scores.Add(score);
db.SaveChanges();
return RedirectToAction("Index");
}
```
5. 添加视图
在 Views 文件夹中添加一个名为 `Index.cshtml` 的视图,用于显示学生列表和成绩列表。
```
@model IEnumerable<StudentScore.Models.Student>
@{
ViewBag.Title = "学生考试成绩管理系统";
}
<h2>学生列表</h2>
<table class="table">
<thead>
<tr>
<th>ID</th>
<th>姓名</th>
<th>班级</th>
</tr>
</thead>
<tbody>
@foreach (var student in ViewBag.Students)
{
<tr>
<td>@student.Id</td>
<td>@student.Name</td>
<td>@student.Class</td>
</tr>
}
</tbody>
</table>
<h2>成绩列表</h2>
<table class="table">
<thead>
<tr>
<th>ID</th>
<th>学生ID</th>
<th>科目</th>
<th>成绩</th>
</tr>
</thead>
<tbody>
@foreach (var score in ViewBag.Scores)
{
<tr>
<td>@score.Id</td>
<td>@score.StudentId</td>
<td>@score.Subject</td>
<td>@score.Score</td>
</tr>
}
</tbody>
</table>
<h2>添加学生</h2>
@using (Html.BeginForm("AddStudent", "Home"))
{
<div class="form-group">
@Html.LabelFor(model => model.Name, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Name, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Name, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Class, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Class, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Class, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" value="添加" class="btn btn-default" />
</div>
</div>
}
<h2>添加成绩</h2>
@using (Html.BeginForm("AddScore", "Home"))
{
<div class="form-group">
@Html.LabelFor(model => model.StudentId, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.DropDownListFor(model => model.StudentId, new SelectList(ViewBag.Students, "Id", "Name"), new { @class = "form-control" })
@Html.ValidationMessageFor(model => model.StudentId, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Subject, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Subject, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Subject, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Score, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Score, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Score, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" value="添加" class="btn btn-default" />
</div>
</div>
}
```
6. 运行项目
在浏览器中输入 `http://localhost:port/Home/Index` 即可访问学生考试成绩管理系统。可以在系统中添加、编辑和删除学生信息和成绩信息。
数据库名称: studentinfo 1. 实现flask框架环境搭建;(30分) 2. 创建model模型数据生成数据库;(25分) 3. 实现学生信息的查询功能;(15分) 4. 实现学生信息的添加功能;(15分) 5. 实现学生信息的删除功能;(15分)
在使用Flask框架进行学生信息管理系统开发过程中,你可以按照以下步骤进行:
1. **环境搭建** (30分):
- 安装Python和Flask框架:首先确保你的机器已安装Python,然后通过`pip install flask`命令安装Flask。
- 创建虚拟环境:推荐使用`venv`或`conda`创建一个新的Python环境,以便隔离依赖。
- 设置项目的目录结构,包含app文件夹,其中包含app.py主程序和templates、static目录用于前端页面资源。
2. **Model模型与数据库生成** (25分):
- 在`models.py`模块中,导入SQLAlchemy库,定义StudentInfo模型类,包含属性(如id, name, gender等):
```python
from flask_sqlalchemy import SQLAlchemy
db = SQLAlchemy()
class StudentInfo(db.Model):
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(100))
# ...其他字段...
```
- 初始化db实例并连接数据库(通常是sqlite或MySQL):
```python
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///studentinfo.db'
db.init_app(app)
```
- 使用`db.create_all()`在运行时自动创建数据库和相关表。
3. **查询功能实现** (15分):
- 在`views.py`里,创建一个路由处理GET请求,接收查询参数,使用ORM查询数据库并返回结果:
```python
@app.route('/search/<int:student_id>')
def search_student(student_id):
student = StudentInfo.query.get(student_id)
return render_template('search_result.html', student=student)
```
4. **添加功能实现** (15分):
- 创建一个POST路由接受表单提交的数据,对新数据进行验证后保存到数据库:
```python
@app.route('/add_student', methods=['POST'])
def add_student():
new_student = StudentInfo(name=request.form['name'], ...)
db.session.add(new_student)
db.session.commit()
return redirect(url_for('index'))
```
5. **删除功能实现** (15分):
- 创建一个路由处理DELETE请求,接收学生ID,从数据库中删除对应记录:
```python
@app.route('/delete/<int:student_id>', methods=['DELETE'])
def delete_student(student_id):
student = StudentInfo.query.get(student_id)
db.session.delete(student)
db.session.commit()
return jsonify({'message': 'Student deleted successfully'})
```
记得在每个功能完成后,别忘了测试,确保各项功能正常工作。
阅读全文
相关推荐
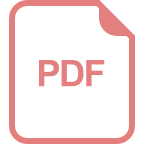
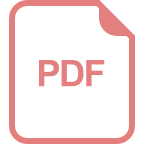
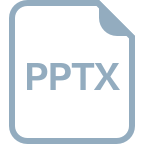













