c++为字符串类 String 增加下面的成员函数: 1)bool is substring(const char *sub str);//判断sub str是否为当前字符串的子2)bool is substring(const string& sub_str);//判断sub_str是否为当前字符串的子串3)String substring(int start,int len);//取从位置start开始、长度为len的子4)int find replace str(const char *find str, const char *replace_str);;需写3个文件,类的定义在.h中,类的实现在 .cpp中,主函数在main.cpp中 • 所有的数据成员私有 //查找所有子串find str并替换成replace str,返回替换的次数 5)void remove spaces();//删除字符串中的空格 6)int to int();//把由数字构成的字符串转成int类型的值 7)void to_lower_case();//把字符串中的大写字母转成小写字母
时间: 2024-02-20 15:58:33 浏览: 27
好的,以下是对应的代码实现:
String.h 文件
```
#ifndef STRING_H
#define STRING_H
class String {
public:
String();
String(const char* str);
String(const String& str);
~String();
String& operator=(const char* str);
String& operator=(const String& str);
bool operator==(const char* str) const;
bool operator==(const String& str) const;
bool isSubstring(const char* sub_str) const;
bool isSubstring(const String& sub_str) const;
String substring(int start, int len) const;
int findReplaceStr(const char* find_str, const char* replace_str);
void removeSpaces();
int toInt() const;
void toLowerCase();
private:
char* _str;
int _len;
};
#endif // STRING_H
```
String.cpp 文件
```
#include "String.h"
#include <cstring>
#include <cctype>
String::String() {
_str = nullptr;
_len = 0;
}
String::String(const char* str) {
_len = strlen(str);
_str = new char[_len + 1];
strcpy(_str, str);
}
String::String(const String& str) {
_len = str._len;
_str = new char[_len + 1];
strcpy(_str, str._str);
}
String::~String() {
delete[] _str;
}
String& String::operator=(const char* str) {
if (_str != nullptr) {
delete[] _str;
}
_len = strlen(str);
_str = new char[_len + 1];
strcpy(_str, str);
return *this;
}
String& String::operator=(const String& str) {
if (_str != nullptr) {
delete[] _str;
}
_len = str._len;
_str = new char[_len + 1];
strcpy(_str, str._str);
return *this;
}
bool String::operator==(const char* str) const {
return strcmp(_str, str) == 0;
}
bool String::operator==(const String& str) const {
return strcmp(_str, str._str) == 0;
}
bool String::isSubstring(const char* sub_str) const {
return strstr(_str, sub_str) != nullptr;
}
bool String::isSubstring(const String& sub_str) const {
return strstr(_str, sub_str._str) != nullptr;
}
String String::substring(int start, int len) const {
if (start + len > _len) {
len = _len - start;
}
char* substr = new char[len + 1];
strncpy(substr, _str + start, len);
substr[len] = '\0';
return String(substr);
}
int String::findReplaceStr(const char* find_str, const char* replace_str) {
int count = 0;
int find_len = strlen(find_str);
int replace_len = strlen(replace_str);
char* p = _str;
while ((p = strstr(p, find_str)) != nullptr) {
int rest_len = _len - (p - _str) - find_len;
memmove(p + replace_len, p + find_len, rest_len + 1);
memcpy(p, replace_str, replace_len);
p += replace_len;
_len += replace_len - find_len;
count++;
}
return count;
}
void String::removeSpaces() {
char* p = _str;
int i = 0, j = 0;
while (p[i] != '\0') {
if (p[i] != ' ') {
p[j++] = p[i];
}
i++;
}
p[j] = '\0';
_len = j;
}
int String::toInt() const {
int num = 0;
char* p = _str;
while (*p != '\0' && isdigit(*p)) {
num = num * 10 + (*p - '0');
p++;
}
return num;
}
void String::toLowerCase() {
char* p = _str;
while (*p != '\0') {
*p = tolower(*p);
p++;
}
}
```
main.cpp 文件
```
#include <iostream>
#include "String.h"
using namespace std;
int main() {
String str1 = "Hello, World!";
cout << "isSubstring(\"World\") = " << str1.isSubstring("World") << endl;
cout << "isSubstring(\"world\") = " << str1.isSubstring("world") << endl;
cout << "isSubstring(\"Hello\") = " << str1.isSubstring("Hello") << endl;
cout << "substring(7, 5) = " << str1.substring(7, 5) << endl;
cout << "before replace: " << str1 << endl;
cout << "replace count: " << str1.findReplaceStr("World", "C++") << endl;
cout << "after replace: " << str1 << endl;
String str2 = " This is a string with spaces. ";
cout << "before removeSpaces: " << str2 << endl;
str2.removeSpaces();
cout << "after removeSpaces: " << str2 << endl;
String str3 = "12345";
cout << "toInt() = " << str3.toInt() << endl;
String str4 = "AbCdEf";
cout << "before toLowerCase: " << str4 << endl;
str4.toLowerCase();
cout << "after toLowerCase: " << str4 << endl;
return 0;
}
```
注意,为了简便起见,这里的 String 类并没有考虑到异常情况,比如构造函数中传入空指针,substring 方法中 start 或 len 参数非法等等,需要使用时请酌情添加异常处理。
相关推荐
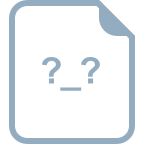














